ScatterCtrl Demo
Scatter series in GUI applications
ScatterCtrl_Demo package does a visual review of ScatterCtrl class features.
Here there are some screenshots:
General view
Demo program contains control samples in different tabs.
All of them have different export possibilities like:
Print Preview
Export as PNG
Export as JPEG
Export as EMF
Copy to Clipboard
Scatter controls lets to export in more formats.
In addition there are different painting modes to let either smoother or faster plots.
|
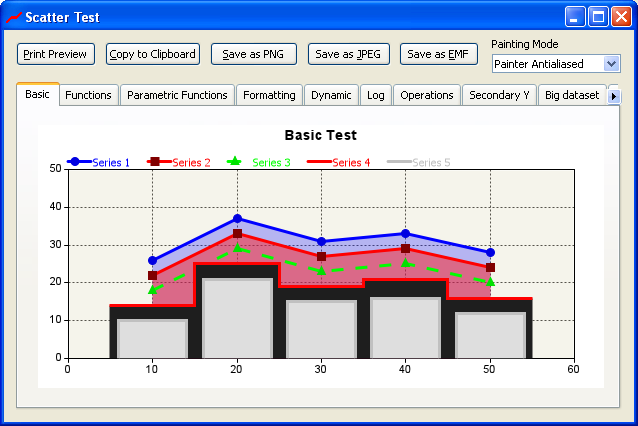
|
|
|
Basic Test
Scatter lets to show different data series changing various parameters like color, line width, dot style.
|
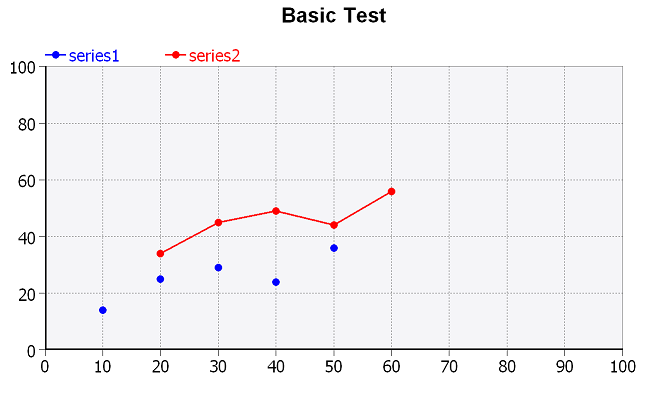
|
|
|
Functions
Using PlotFunction() it is possible to directly draw functions instead of just stored data.
|
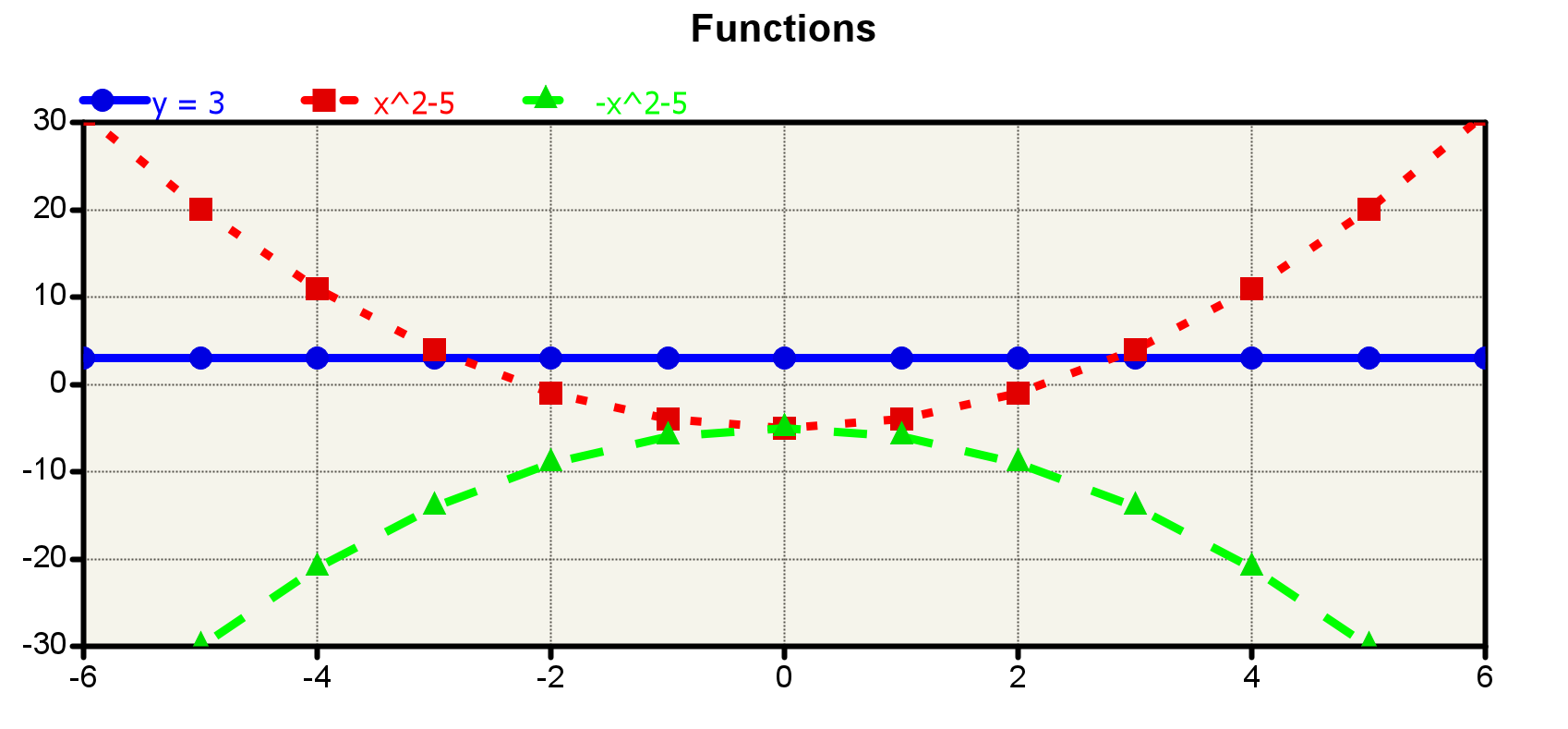
|
|
|
Parametric Functions
While PlotFunction() functions just return the vertical axis, PlotParaFunction() functions return the XY coordinates of every point.
This options lets full freedom inserting XY series.
|
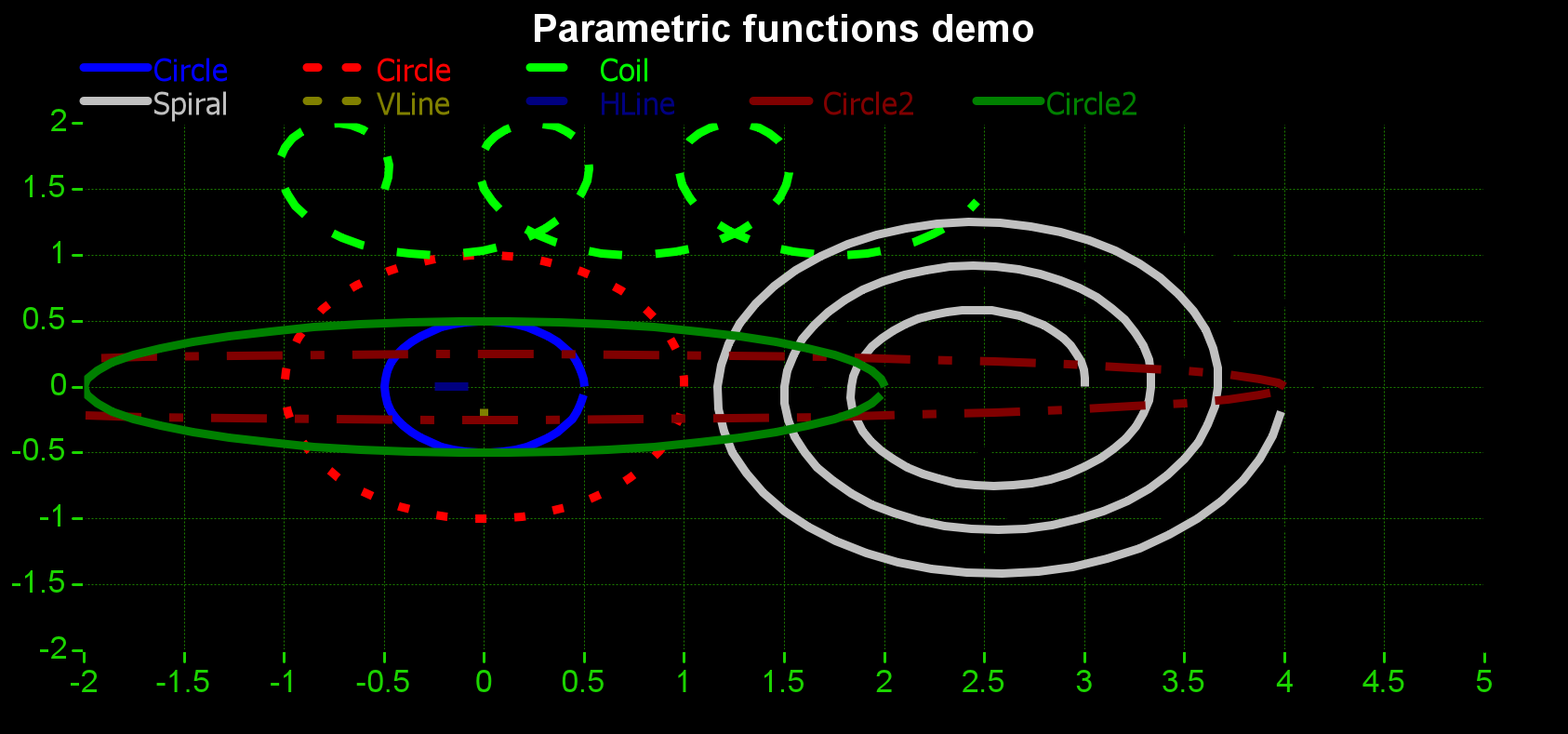
|
|
|
Formatting
ScatterDraw/ScatterCtrl lets to insert X and Y labels, XY scaling and range, point format and size and standard or user defined axis labels formating.
In addition SetMouseHandling(true) lets to use the mouse to zoom and move the graphs in X axis.
|
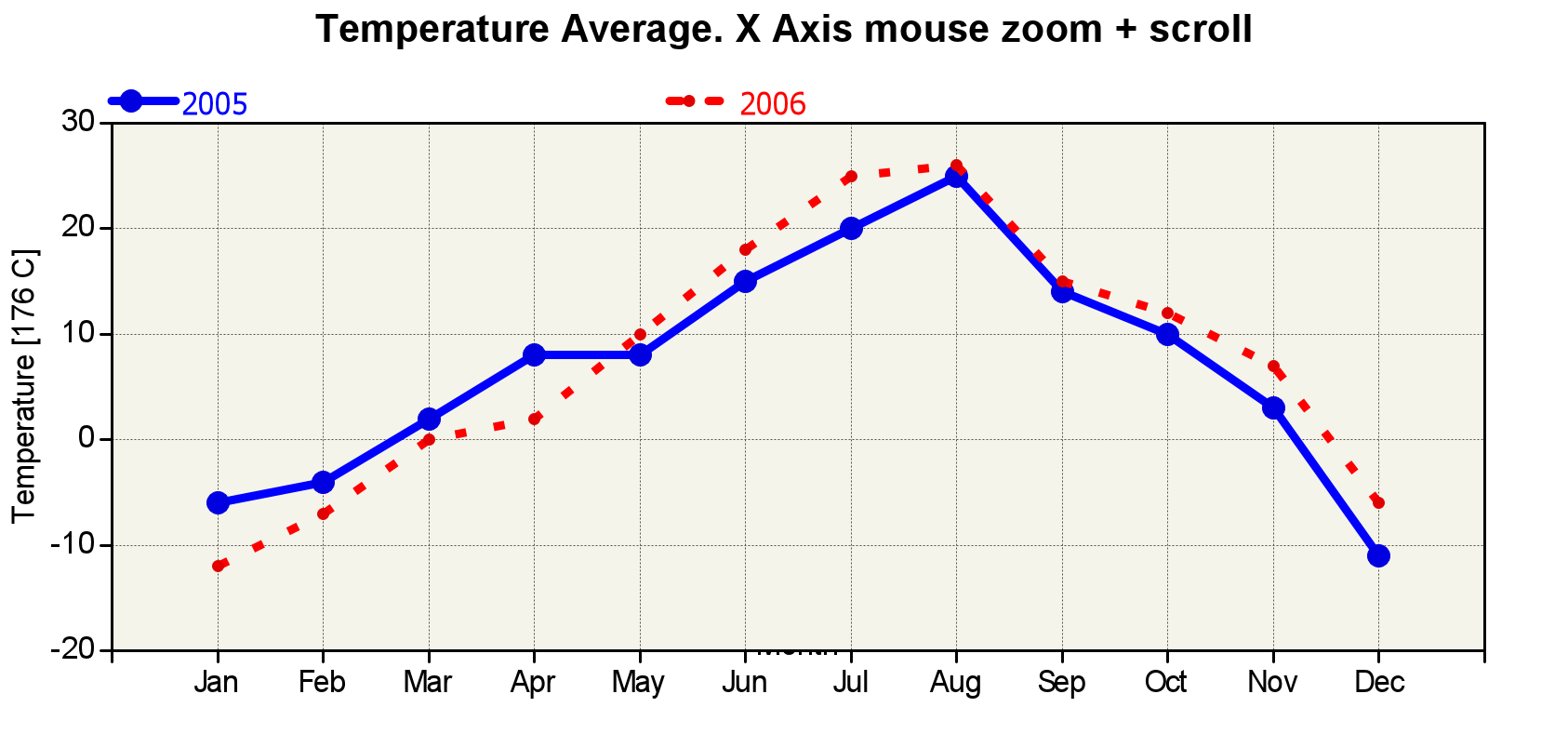
|
|
|
Dynamic
ScatterCtrl is not an static control and easily lets to include dynamic series handling.
|
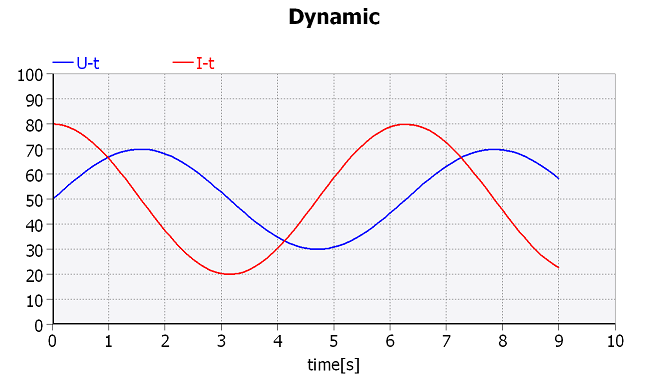
|
|
|
Log
Versatile axis scaling and labeling through cbModifFormatX and cbModifFormatY lets to include not only time and date axis but also logarithmic ones.
|
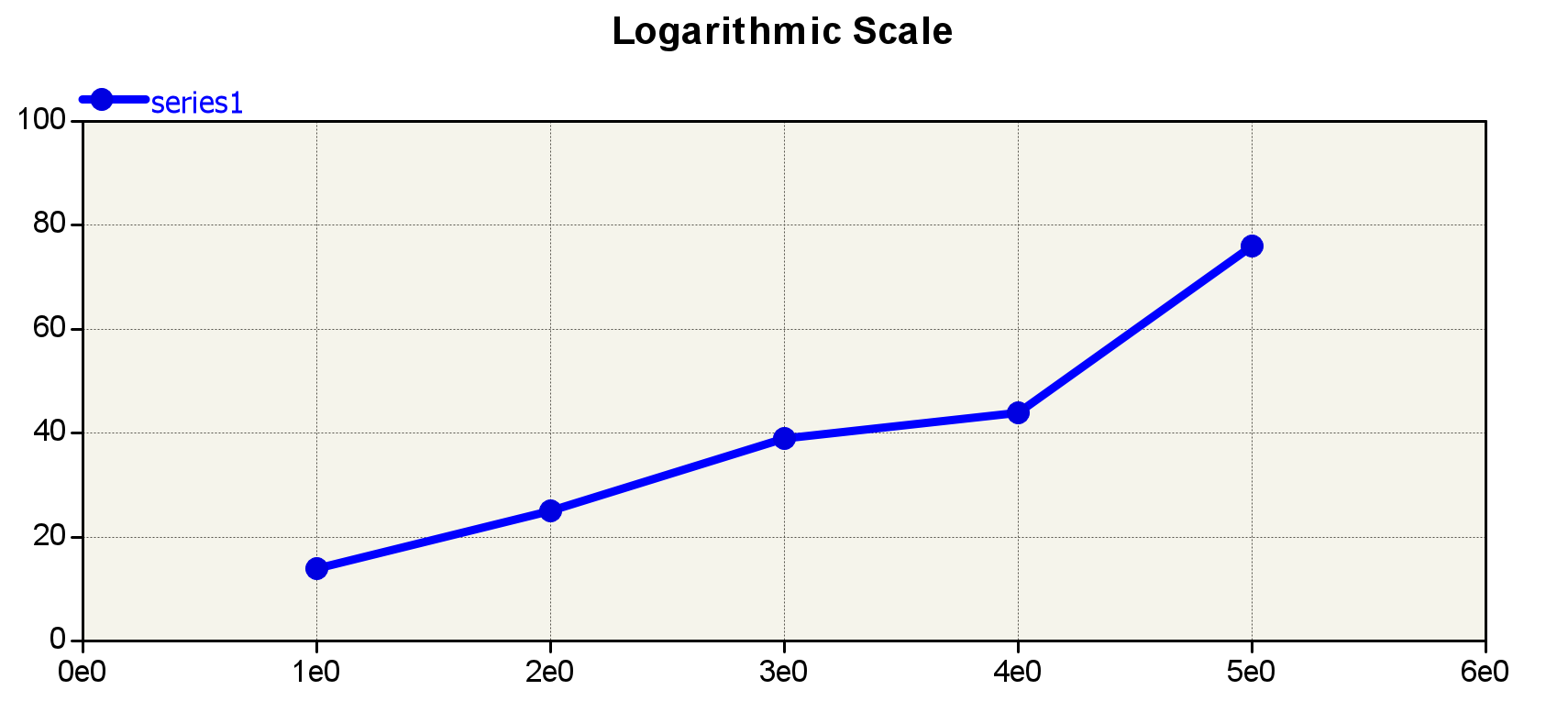
|
|
|
Operations
Series dynamic handling is easy. This demo lets the user to add and remove series.
|
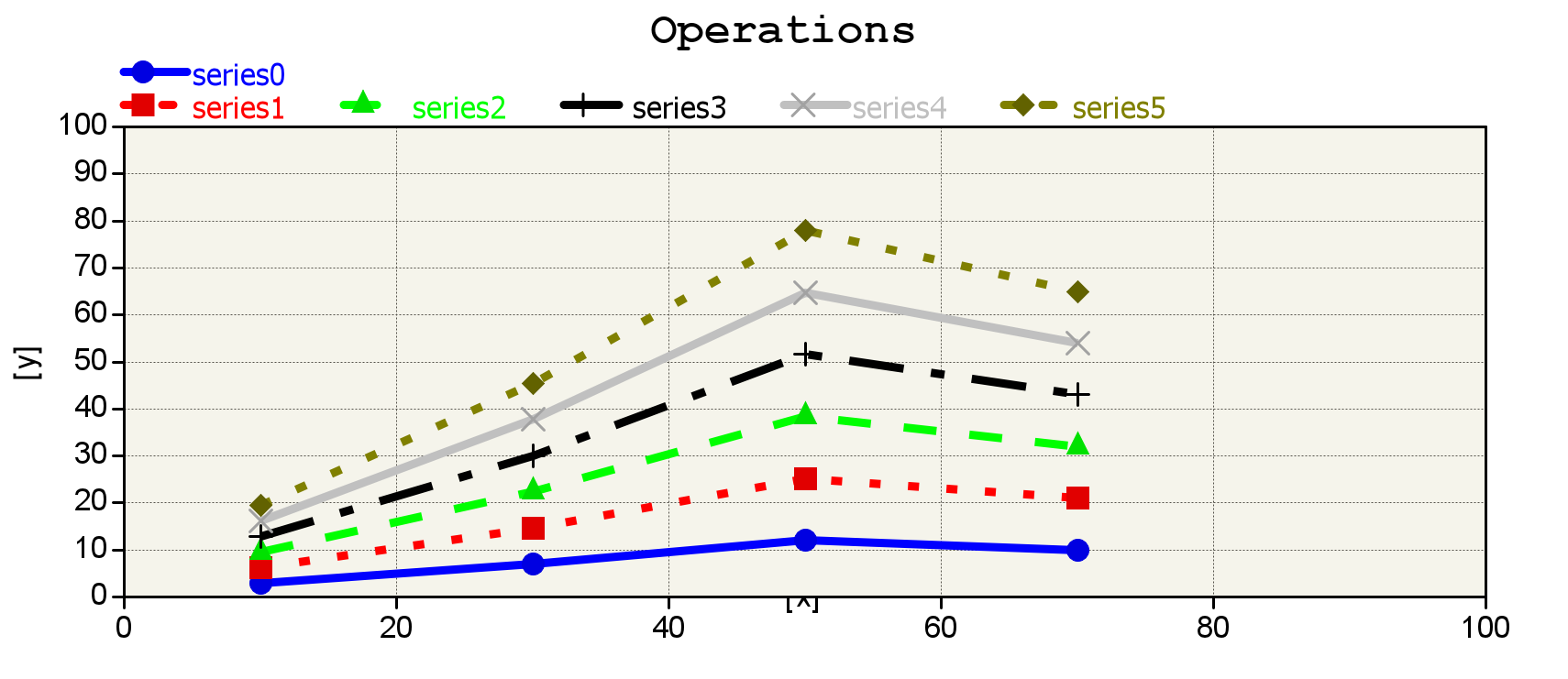
|
|
|
Secondary Y axis
It is possible to add a secondary right side vertical Y axis and scale data series to it.
In addition SetMouseHandling(true, true) lets to use the mouse to zoom and move the graphs in X and Y axis.
|
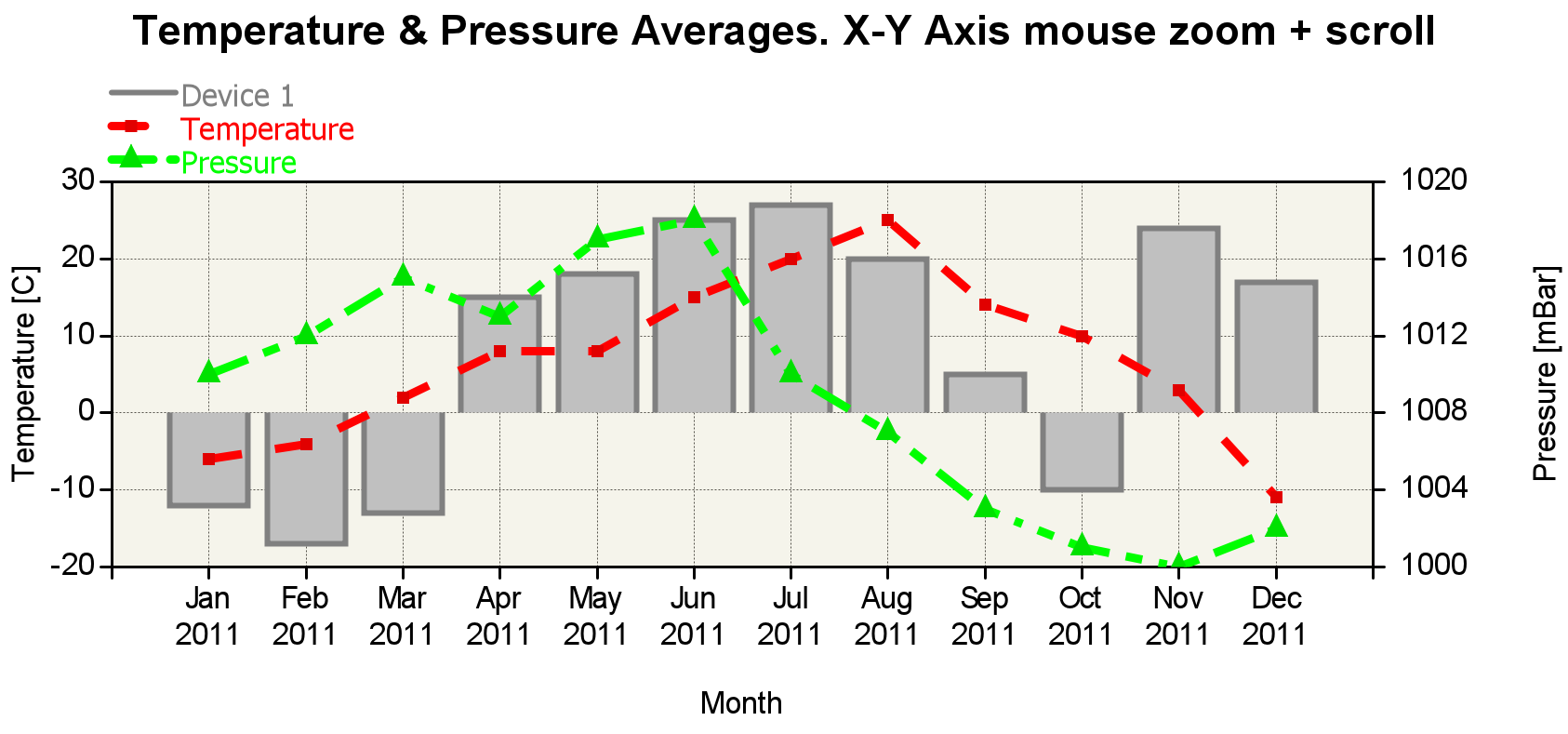
|
|
|
Big datasets
Big datasets are hard to handle. However there are two functions that speed up dramatically ScatterDraw/ScatterCtrl response in X axis sequential datasets like time series:
SetSequentialX: Indicates that the data has been sequentially inserted following X axis
SetFastViewX: Indicates that it will be viewed a point per horizontal pixel. This point will be the average of all data that is in that pixel
|
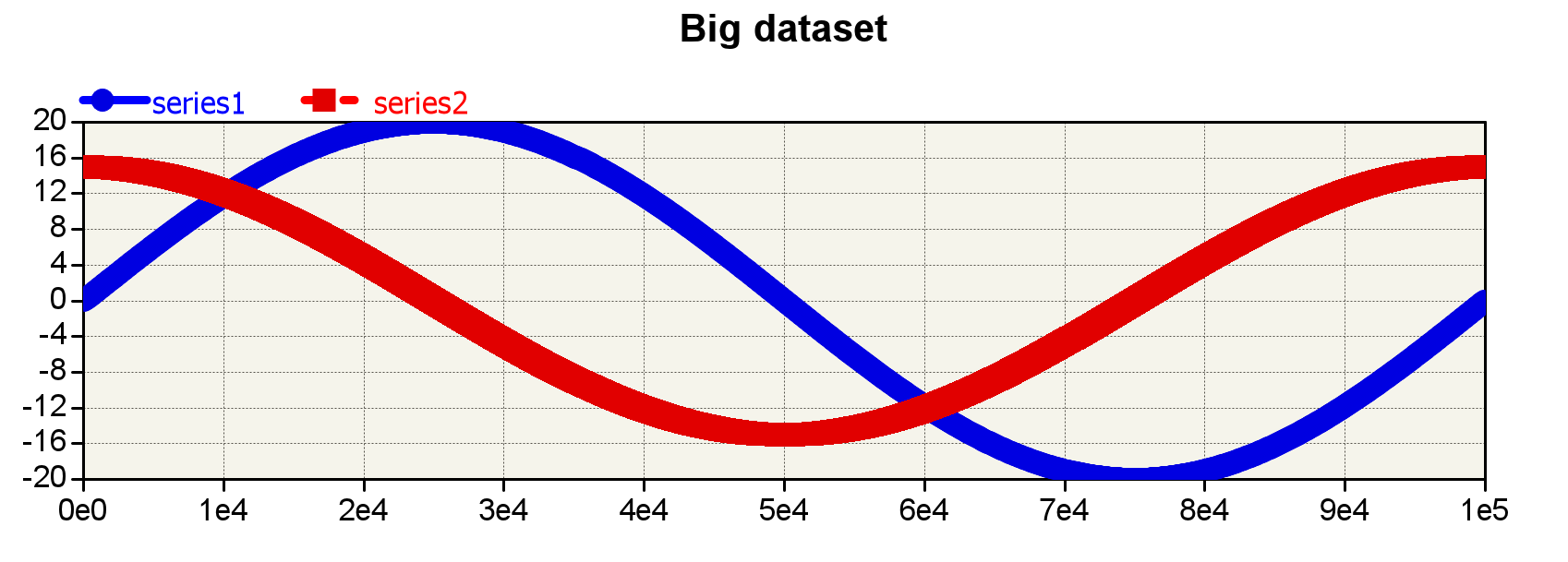
|
|
|
User graph
User can set custom graph types and marks.
|
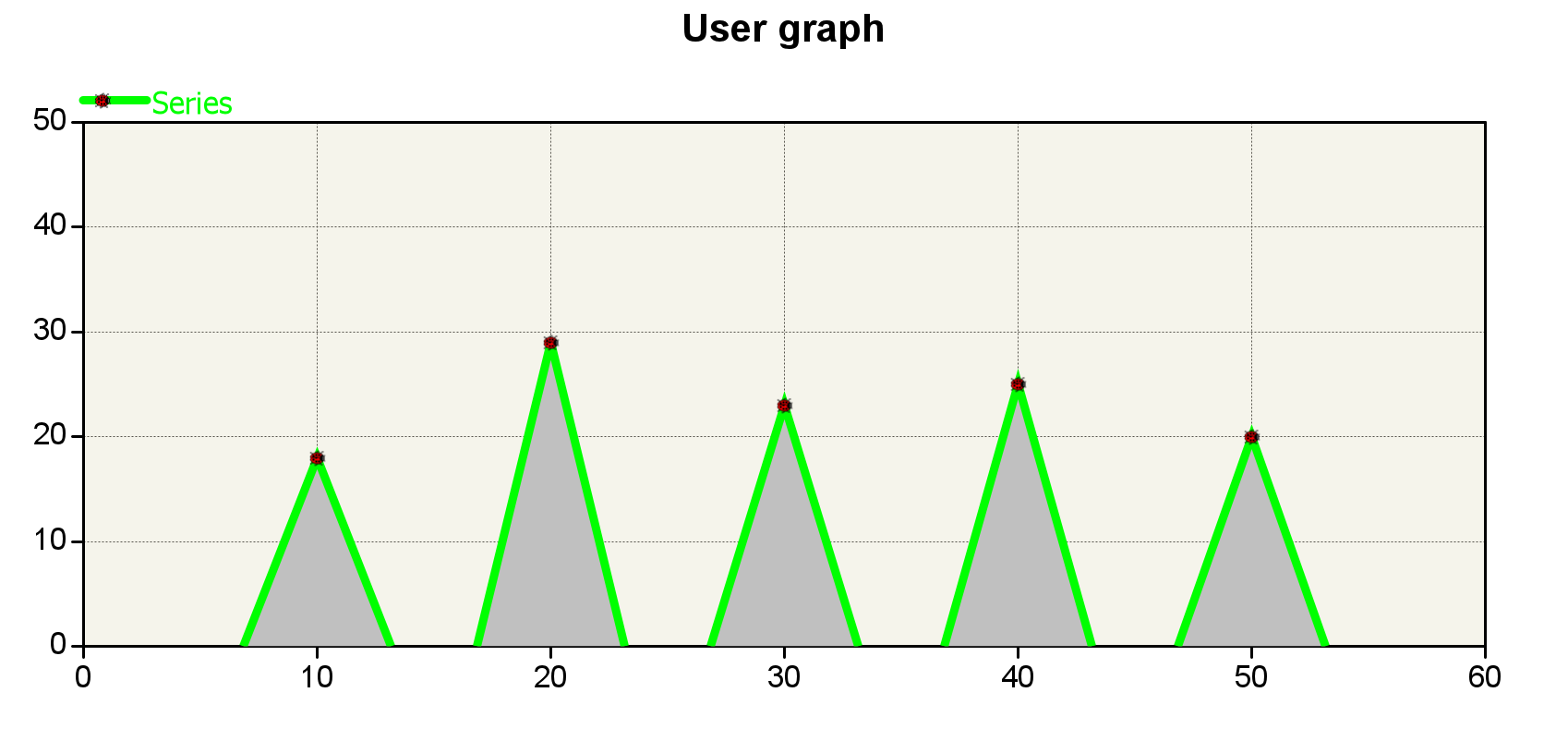
|
|
|
ScatterCtrl_Demo.h
#ifndef _ScatterCtrl_Demo_ScatterCtrl_Demo_h
#define _ScatterCtrl_Demo_ScatterCtrl_Demo_h
#include <ScatterCtrl/ScatterCtrl.h>
#include <ScatterCtrl/PieCtrl.h>
using namespace Upp;
#define LAYOUTFILE <ScatterCtrl_Demo/ScatterCtrl_Demo.lay>
#include <CtrlCore/lay.h>
#define IMAGECLASS MyImages
#define IMAGEFILE <ScatterCtrl_Demo/ScatterCtrl_Demo.iml>
#include <Draw/iml_header.h>
struct ScatterDemo : StaticRect {
virtual void Init() {};
virtual ScatterCtrl &Scatter() = 0;
};
void RegisterExample(const char *name, ScatterDemo* (*ctrl)(), String fileName);
class Tab1_Basic : public WithTab1Basic<ScatterDemo> {
public:
typedef Tab1_Basic CLASSNAME;
virtual void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Pointf> sDemoRed1, sDemoRed2,sDemoGreen;
Vector<Pointf> s1;
double s2y[5];
double s3x[5], s3y[5];
Vector<double> s4x, s4y;
VectorMap<double, double> s5;
};
class Tab2_Functions : public WithTab2Functions<ScatterDemo> {
public:
typedef Tab2_Functions CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
class MySource : public FuncSource {
public:
void Init(double data) {
this->data = data;
function = [=](double x) {return Calc(x);};
}
double Calc(double x);
private:
double data;
};
MySource source;
};
class Tab3_ParametricFunctions : public WithTab3ParametricFunctions<ScatterDemo> {
public:
typedef Tab3_ParametricFunctions CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
};
class Tab4_Formatting : public WithTab4Formatting<ScatterDemo> {
public:
typedef Tab4_Formatting CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Pointf> s1, s2;
};
class Tab5_Dynamic : public WithTab5Dynamic<ScatterDemo> {
public:
typedef Tab5_Dynamic CLASSNAME;
void Init();
void Animate();
void Start();
void Stop();
void Reset();
void PgDown();
void PgUp();
void Plus();
void Minus();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
double t;
Vector<Pointf> s1, s2;
};
class Tab6_Logarithmic : public WithTab6Logarithmic<ScatterDemo> {
public:
typedef Tab6_Logarithmic CLASSNAME;
void Init();
void FormatX(String& s, int i, double d);
void FormatXGridUnits(String& s, int i, double d);
void SetGridLinesX(Vector<double>& unitsX);
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Pointf> s1;
};
class Tab7_Operations : public WithTab7Operations<ScatterDemo> {
public:
typedef Tab7_Operations CLASSNAME;
void Init();
void AddSeries();
void RemoveFirstSeries();
void RemoveLastSeries();
void RemoveAllSeries();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Upp::Array<Vector<Pointf> > series;
};
class Tab8_Secondary : public WithTab8Secondary<ScatterDemo> {
public:
typedef Tab8_Secondary CLASSNAME;
void Init();
void MyFormat(String& s, int , double d)
{
s = Format("%Mon", fround(d));
if (!s.IsEmpty())
s << "\n2011";
}
void MyFormatDelta(String& s, int , double d)
{
s = FormatDouble(d, 1) + " " + t_("months");
}
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Pointf> s1, s2, s3;
};
class Tab9_Big : public WithTab9Big<ScatterDemo> {
public:
typedef Tab9_Big CLASSNAME;
void Init();
void OnFastView() {scatter.SetFastViewX(fastView);};
void OnSequentialX() {scatter.SetSequentialXAll(sequentialX);};
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Pointf> s1,s2;
};
class Tab10_User : public WithTab10User<ScatterDemo> {
public:
typedef Tab10_User CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
double sx[5], sy[5];
};
class Tab11_Trend : public WithTab11Trend<ScatterDemo> {
public:
typedef Tab11_Trend CLASSNAME;
Tab11_Trend() {};
void Init();
void OnSeries();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<double> s1x, s1y;
Vector<double> s2x, s2y;
LinearEquation linear;
PolynomialEquation poly2, poly4;
FourierEquation fourier;
ExponentialEquation exponential;
Rational1Equation rational1;
SinEquation sin;
};
class Tab12_Linked : public WithTab12Linked<ScatterDemo> {
public:
typedef Tab12_Linked CLASSNAME;
virtual void Init();
virtual ScatterCtrl &Scatter() {return scatter1;};
private:
Vector<Pointf> s1, s2, s3;
void OnLink();
};
class TabUserEquation : public WithTabUserEquation<ScatterDemo> {
public:
typedef TabUserEquation CLASSNAME;
virtual void Init();
void OnUpdate();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
UserEquation userEquation;
};
class TabRangePlot : public WithTabRangePlot<ScatterDemo> {
public:
typedef TabRangePlot CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Vector <double> > s1, s2;
Vector<int> idsRange;
Vector<Pointf> sdata, smin, smax;
VectorPointf dmin, dmax;
DataRange range;
};
class TabStackedPlot : public WithTabStackedPlot<ScatterDemo> {
public:
typedef TabStackedPlot CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
void OnPlot();
private:
Vector<Pointf> s1, s2, s3;
VectorPointf ds1, ds2, ds3;
DataStackedY stacked;
};
class TabBubblePlot : public WithTabBubblePlot<ScatterDemo> {
public:
typedef TabBubblePlot CLASSNAME;
void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
private:
Vector<Vector <double> > s1;
Vector<int> idsBubble;
Vector<String> labels;
};
class Tab_UserPaint : public WithTabUserPaint<ScatterDemo> {
public:
typedef Tab_UserPaint CLASSNAME;
virtual void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
void OnPainter(Painter &w) {OnPaint(w);}
void OnDraw(Draw &w) {OnPaint(w);}
template <class T>
void OnPaint(T& w) {
int plotW = scatter.GetPlotWidth(), plotH = scatter.GetPlotHeight();
DrawLineOpa(w, 0, 0, plotW, plotH, 1, 1, 1, LtRed(), "2 2");
DrawText(w, plotW/5, plotH/5, 0, "Fixed line", Arial(15), Black());
String text = "Fixed text";
Font font = Arial(30);
Size sz = GetTextSizeSpace(text, font);
FillRectangleOpa(w, plotW - sz.cx - 10, 10, plotW - 10, 10 + sz.cy, 0.3, Null, LtGreen());
DrawText(w, plotW - sz.cx - 10, 10, 0, text, font, Black());
if (scatter.GetXMin() < 1 && scatter.GetXMax() > 1) {
Pointf p;
for (int i = 0; i < s1.GetCount(); ++i) {
if (s1[i].x >= 1) {
p = s1[i];
break;
}
}
if (scatter.GetYMin() < p.y && scatter.GetYMax() > p.y) {
double posx = scatter.GetPosX(p.x);
double posy = scatter.GetPosY(p.y);
double sizex = scatter.GetSizeX(0.2);
FillRectangleOpa(w, posx - sizex/2., posy - sizex/2.,
posx + sizex/2., posy + sizex/2.,
0.8, Null, LtGreen());
Vector<Pointf> s;
s << Pointf(posx - sizex/2., posy - sizex/2.)
<< Pointf(posx + sizex/2., posy - sizex/2.)
<< Pointf(posx + sizex/2., posy + sizex/2.)
<< Pointf(posx - sizex/2., posy + sizex/2.)
<< Pointf(posx - sizex/2., posy - sizex/2.);
DrawPolylineOpa(w, s, 1, 1, 2, Green(), "", Null);
DrawText(w, posx + sizex/2., posy, 0, Format("Pos %.2f, %.2f fixed font", p.x, p.y), Arial(15), Black());
double sizeT = scatter.GetSizeY(0.05);
Font font = Arial((int)sizeT);
DrawText(w, posx + sizex/2., posy + 15, 0, Format("Pos %.2f, %.2f scaled font", p.x, p.y), font, Black());
}
}
}
private:
Vector<Pointf> s1;
};
class Tab19_Surf : public WithTabSurf<ScatterDemo> {
public:
typedef Tab19_Surf CLASSNAME;
virtual void Init();
virtual ScatterCtrl &Scatter() {return scatter;};
void OnChange();
void Init_DatasetSimple();
void Init_Dataset();
void Init_DataExplicit();
private:
ExplicitData funData;
TableDataVector data;
TableDataVector dataFun;
Vector<double> zData, xAxis, yAxis;
Vector<double> zDataFun, xAxisFun, yAxisFun;
Vector<Pointf> isolines, numbersPos;
Vector<String> labels;
};
class TabPie : public WithTabPie<StaticRect> {
public:
typedef TabPie CLASSNAME;
void Init();
};
class ScatterCtrl_Demo : public WithScatterCtrl_Demo<TopWindow> {
typedef ScatterCtrl_Demo CLASSNAME;
public:
ScatterCtrl_Demo();
TabPie tabPie;
private:
void Preview();
void SavePNG();
void SaveJPG();
#ifdef PLATFORM_WIN32
void SaveEMF();
#endif
void CopyClipboard();
void SetMode();
void OnSel();
Vector<StaticRect *> examplesRects;
};
#endif
tab14_UserEquation.cpp
#include "ScatterCtrl_Demo.h"
void TabUserEquation::Init()
{
CtrlLayout(*this);
SizePos();
equation <<= "25 + 10*sin(0.5*x + 5)";
fromX <<= 0;
toX <<= 100;
fromY <<= 0;
toY <<= 50;
update.WhenAction = THISBACK(OnUpdate);
scatter.SetMouseHandling(true, true).ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
OnUpdate();
}
void TabUserEquation::OnUpdate()
{
if (fromX >= toX || fromY >= toY) {
Exclamation("Wrong limits");
return;
}
userEquation.Init("User equation", ~equation);
scatter.RemoveAllSeries();
scatter.AddSeries(userEquation).Legend(userEquation.GetFullName()).NoMark().Stroke(2).Units("m3/s");
scatter.SetXYMin(fromX, fromY);
scatter.SetRange(toX - fromX, toY - fromY);
scatter.ZoomToFit(true, true);
}
ScatterDemo *ConstructUserEquation()
{
static TabUserEquation tab;
return &tab;
}
INITBLOCK {
RegisterExample("User equation", ConstructUserEquation, __FILE__);
}
tab13.cpp
#include "ScatterCtrl_Demo.h"
void Tab12::Init()
{
CtrlLayout(*this);
HSizePos().VSizePos();
s1 << Pointf(50, 10) << Pointf(100, 20) << Pointf(150, 30) << Pointf(200, 20) << Pointf(250, 10);
scatter.AddSeries(s1).Legend("Series 1").Fill();
scatter.SetXYMin(50, 0).SetRange(200, 40).SetMajorUnits(50, 10).SetPolar();
scatter.ShowInfo().ShowContextMenu().ShowPropertiesDlg().SetPopText("h", "v", "v2").SetMouseHandling(true, true);
}
ScatterDemo *Construct12()
{
static Tab12 tab;
return &tab;
}
INITBLOCK {
RegisterExample("Polar plot", Construct12, __FILE__);
}
main.cpp
#include "ScatterCtrl_Demo.h"
#include <Report/Report.h>
#include <PdfDraw/PdfDraw.h>
#define IMAGECLASS MyImages
#define IMAGEFILE <ScatterCtrl_Demo/ScatterCtrl_Demo.iml>
#include <Draw/iml_source.h>
struct Example {
ScatterDemo* (*ctrl)();
String name;
int index;
};
Upp::Array<Example>& Examples()
{
static Upp::Array<Example> x;
return x;
}
void RegisterExample(const char *name, ScatterDemo* (*ctrl)(), String fileName)
{
Example& x = Examples().Add();
x.name = name;
x.ctrl = ctrl;
x.index = ScanInt(GetFileName(fileName).Mid(3, 2));
}
bool CompareExamples(Example &a, Example &b) {return a.index < b.index;}
GUI_APP_MAIN
{
Sort(Examples(), CompareExamples);
ScatterCtrl_Demo().Run();
}
ScatterCtrl_Demo::ScatterCtrl_Demo()
{
CtrlLayout(*this, "Scatter Test");
for (int i = 0; i < Examples().GetCount(); ++i)
Examples()[i].ctrl()->Init();
examplesList.NoHorzGrid().NoVertGrid();
examplesList.AddColumn("Example name");
for (int i = 0; i < Examples().GetCount(); ++i) {
examplesList.Add(Examples()[i].name);
Add((*(Examples()[i].ctrl())).HSizePosZ(180, 4).VSizePosZ(4, 8));
examplesRects.Add(Examples()[i].ctrl());
}
examplesList.Add("Pie chart");
tabPie.Init();
Add(tabPie.HSizePosZ(180, 4).VSizePosZ(4, 8));
examplesRects.Add(&tabPie);
for (int i = 0; i < examplesRects.GetCount(); ++i)
examplesRects[i]->Hide();
bPreview <<= THISBACK(Preview);
bSavePNG <<= THISBACK(SavePNG);
bSaveJPG <<= THISBACK(SaveJPG);
#ifdef PLATFORM_WIN32
bSaveEMF <<= THISBACK(SaveEMF);
#else
bSaveEMF.Hide();
#endif
bCopyClipboard <<= THISBACK(CopyClipboard);
paintMode.Add(ScatterDraw::MD_ANTIALIASED, "Painter Antialiased")
.Add(ScatterDraw::MD_NOAA, "Painter No-Antialiased")
.Add(ScatterDraw::MD_SUBPIXEL, "Painter Subpixel")
.Add(ScatterDraw::MD_DRAW, "Draw");
paintMode <<= THISBACK(SetMode);
paintMode.SetData(ScatterDraw::MD_ANTIALIASED);
SetMode();
examplesList.WhenSel = THISBACK(OnSel);
examplesList.SetCursor(17);
OnSel();
Sizeable().Zoomable().Icon(MyImages::i1());
}
void ScatterCtrl_Demo::OnSel()
{
for (int i = 0; i < examplesRects.GetCount(); ++i)
examplesRects[i]->Hide();
if (examplesList.GetCursor() < 0)
return;
examplesRects[examplesList.GetCursor()]->Show();
}
void ScatterCtrl_Demo::Preview()
{
Report r;
r.Landscape();
Size pageSize = r.GetPageSize();
const Drawing &w = Examples()[examplesList.GetCursor()].ctrl()->Scatter().GetDrawing();
r.DrawDrawing(0, 0, pageSize.cx, pageSize.cy, w);
Perform(r);
}
void ScatterCtrl_Demo::SavePNG()
{
int ntab = examplesList.GetCursor();
Examples()[ntab].ctrl()->Scatter().SaveToFile(AppendFileName(GetDesktopFolder(), Format("scatter%d.png", ntab)));
}
void ScatterCtrl_Demo::SaveJPG()
{
int ntab = examplesList.GetCursor();
Examples()[ntab].ctrl()->Scatter().SaveToFile(AppendFileName(GetDesktopFolder(), Format("scatter%d.jpg", ntab)));
}
#ifdef PLATFORM_WIN32
void ScatterCtrl_Demo::SaveEMF()
{
int ntab = examplesList.GetCursor();
Examples()[ntab].ctrl()->Scatter().SaveAsMetafile(AppendFileName(GetDesktopFolder(), Format("scatter%d.emf", ntab)));
}
#endif
void ScatterCtrl_Demo::CopyClipboard()
{
Examples()[examplesList.GetCursor()].ctrl()->Scatter().SaveToClipboard();
}
void ScatterCtrl_Demo::SetMode()
{
for (int i = 0; i < Examples().GetCount(); ++i)
Examples()[i].ctrl()->Scatter().SetMode(~paintMode);
}
tab17_BubblePlot.cpp
#include "ScatterCtrl_Demo.h"
void TabBubblePlot::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetMouseHandling(true, true).ShowContextMenu();
for (double size = 0; size <= 10; size += 2.) {
Vector<double> &data = s1.Add();
double x = size;
double y = (10 + 10*Random(30)*size);
double diameter = Random(80);
data << x << y << diameter;
labels << Format("%.0f cm", diameter);
}
idsBubble << 2;
static Vector<int> idVoid;
scatter.AddSeries(s1, 0, 1, idVoid, idVoid, idsBubble).Legend("Importance").MarkStyle<BubblePlot>()
.MarkColor(Green()).MarkBorderColor(LtRed()).MarkBorderWidth(3)
.AddLabelSeries(labels, 0, 0, StdFont().Height(15).Bold(), ALIGN_CENTER);
scatter.SetLabelY("Size").Responsive();
scatter.ZoomToFit(true, true, 0.2);
}
ScatterDemo *Construct17()
{
static TabBubblePlot tab;
return &tab;
}
INITBLOCK {
RegisterExample("BubblePlot", Construct17, __FILE__);
}
tab1_Basic.cpp
#include "ScatterCtrl_Demo.h"
enum {
SERIE2
};
void Tab1_Basic::Init()
{
CtrlLayout(*this);
SizePos();
s1 << Pointf(12, 26) << Pointf(20, 37) << Pointf(30, 31) << Pointf(40, 33) << Pointf(50, 28);
scatter.AddSeries(s1).Legend("Series 1").Opacity(0.3).Fill().MarkBorderColor();
s2y[0] = 22; s2y[1] = 33; s2y[2] = 27; s2y[3] = 29; s2y[4] = 24;
scatter.AddSeries(s2y, 5, 10, 10).Legend("Series 2").Id(SERIE2).PlotStyle<LineSeriesPlot>()
.Dash("").MarkColor(Red()).Fill().Opacity(0.5).MarkBorderColor();
s3y[0] = 18; s3y[1] = 29; s3y[2] = 23; s3y[3] = 25; s3y[4] = 20;
s3x[0] = 8; s3x[1] = 20; s3x[2] = 30; s3x[3] = 40; s3x[4] = 50;
scatter.AddSeries(s3x, s3y, 5).Legend("Series 3").MarkBorderColor();
s4y << 14 << 25 << 19 << 21 << 16;
s4x << 10 << 20 << 30 << 40 << 50;
scatter.AddSeries(s4x, s4y).Legend("Series 4").PlotStyle<StaggeredSeriesPlot>().Dash("").NoMark().Fill().Stroke(3, LtRed());
s5.Add(10, 10);
s5.Add(20, 21);
s5.Add(30, 15);
s5.Add(40, 16);
s5.Add(50, 12);
int barWidth = 4;
scatter.AddSeries(s5).Legend("Series 5").PlotStyle<BarSeriesPlot>().BarWidth(barWidth).Dash("").NoMark().Fill();
scatter.SetRange(60, 50).SetMajorUnits(10, 10);
scatter.ShowAllMenus().SetPopText("h", "v", "v2").SetMouseHandling(true, true);
scatter.SetLegendPos(Point(20, 20));
}
ScatterDemo *Construct1()
{
static Tab1_Basic tab;
return &tab;
}
INITBLOCK {
RegisterExample("Basic", Construct1, __FILE__);
}
tab12_Linked.cpp
#include "ScatterCtrl_Demo.h"
void Tab12_Linked::Init()
{
CtrlLayout(*this);
SizePos();
scatter1.SetRange(1000, 40).SetXYMin(0, -20);
scatter1.SetMouseHandling(true).SetMaxRange(5000).SetMinRange(2);
scatter2.SetRange(1000, 40).SetXYMin(0, -20);
scatter2.SetMouseHandling(true).SetMaxRange(5000).SetMinRange(2);
scatter3.SetRange(1000, 40).SetXYMin(0, -20);
scatter3.SetMouseHandling(true).SetMaxRange(5000).SetMinRange(2);
for (int t = 0; t < 3000; ++t) {
s1 << Pointf(t, 20*sin(6*M_PI*t/500) + 5*sin(3*M_PI*t/500 + M_PI/3) + 2*sin(14*M_PI*t/500 + M_PI/5));
s2 << Pointf(t, 50*sin(6*1.1*M_PI*t/500));
s3 << Pointf(t, 20*sin(6*1.2*M_PI*t/500));
}
scatter1.AddSeries(s1).Legend("Series 1").NoMark().Stroke(2, LtRed());
scatter2.AddSeries(s2).Legend("Series 2").NoMark().Stroke(2, LtBlue());
scatter3.AddSeries(s3).Legend("Series 3").NoMark().Stroke(2, LtGreen());
scatter1.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
scatter2.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
scatter3.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
link <<= true;
OnLink();
link.WhenAction = THISBACK(OnLink);
}
void Tab12_Linked::OnLink() {
if (link) {
scatter1.SetTitle("Plots are linked together");
scatter2.LinkedWith(scatter1);
scatter3.LinkedWith(scatter1);
} else {
scatter1.SetTitle("Plots are not linked now");
scatter2.Unlinked();
scatter3.Unlinked();
}
}
ScatterDemo *Construct12()
{
static Tab12_Linked tab;
return &tab;
}
INITBLOCK {
RegisterExample("Linked controls", Construct12, __FILE__);
}
tab2_Functions.cpp
#include "ScatterCtrl_Demo.h"
double funct1(double ) {return 3;}
double funct2(double x) {return (x*x-5);}
double funct3(double x) {return (-x*x-5);}
void vfunct1(double& y, double ) {y = 0;}
void vfunct2(double& y, double x) {y = x*x;}
void vfunct3(double& y, double x) {y = -x*x;}
double Tab2_Functions::MySource::Calc(double x) {return x + data/10;}
void Tab2_Functions::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(12, 60).SetXYMin(-6, -30).SetMajorUnits(2, 10);
scatter.SetMouseHandling(true);
scatter.AddSeries(&funct1).Legend("y = 3").NoMark();
scatter.AddSeries(&funct2).Legend("y = x^2-5").NoMark();
scatter.AddSeries(&funct3).Legend("y = -x^2-5").NoMark();
scatter.AddSeries(&vfunct1).Legend("y = 0").NoMark();
scatter.AddSeries(&vfunct2).Legend("y = x^2").NoMark();
scatter.AddSeries(&vfunct3).Legend("y = -x^2").NoMark();
source.Init(23);
scatter.AddSeries(source).Legend("My source").NoMark();
}
ScatterDemo *Construct2()
{
static Tab2_Functions tab;
return &tab;
}
INITBLOCK {
RegisterExample("Explicit Functions", Construct2, __FILE__);
}
tab16_StackedPlot.cpp
#include "ScatterCtrl_Demo.h"
void TabStackedPlot::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetMouseHandling(true, true).ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
for (int x = 0; x <= 10; ++x) {
s1 << Pointf(x, Random(50));
s2 << Pointf(x, Random(50));
s3 << Pointf(x, Random(50));
}
ds1.Init(s1);
ds2.Init(s2);
ds3.Init(s3);
stacked.Add(ds1).Add(ds2).Add(ds3);
scatter.AddSeries(stacked.Get(2)).Legend("s3").Fill();
scatter.AddSeries(stacked.Get(1)).Legend("s2").Fill();
scatter.AddSeries(stacked.Get(0)).Legend("s1").Fill();
scatter.SetLabelY("Data").SetLabelX("time [seg]")
.ShowButtons().Responsive();
type = 0;
type.WhenAction = THISBACK(OnPlot);
OnPlot();
}
void TabStackedPlot::OnPlot()
{
stacked.Set100(type == 1);
scatter.ZoomToFit(true, type != 1, 0.2);
if (type == 1) {
scatter.SetXYMin(-2, -10);
scatter.SetRange(14, 120);
scatter.SetMajorUnits(2, 10);
scatter.SetMinUnits(-2, -10);
} else {
scatter.SetXYMin(-2, Null);
scatter.SetRange(14, Null);
scatter.SetMajorUnits(2, Null);
scatter.SetMinUnits(-2, Null);
}
}
ScatterDemo *Construct16()
{
static TabStackedPlot tab;
return &tab;
}
INITBLOCK {
RegisterExample("StackedPlot", Construct16, __FILE__);
}
tab18_UserPaint.cpp
#include "ScatterCtrl_Demo.h"
void Tab_UserPaint::Init()
{
CtrlLayout(*this);
SizePos();
double a = 1, b = 0, c = 1;
for (double x = -6; x < 6; x += 0.1)
s1 << Pointf(x, a*exp(-sqr(x - b)/(2*c*c)));
scatter.AddSeries(s1).Legend("Gauss").Opacity(0.3).Fill().NoMark();
scatter.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg().
SetMouseHandling(true, true);
scatter.SetLegendPos(Point(20, 20)).SetLegendAnchor(ScatterDraw::RIGHT_BOTTOM);
scatter.Rotate(ScatterCtrl::Angle_0);
scatter.ZoomToFit(true, true);
scatter.WhenPainter = THISBACK(OnPainter);
scatter.WhenDraw = THISBACK(OnDraw);
}
ScatterDemo *Construct18()
{
static Tab_UserPaint tab;
return &tab;
}
INITBLOCK {
RegisterExample("User paint", Construct18, __FILE__);
}
tab5_Dynamic.cpp
#include "ScatterCtrl_Demo.h"
void Tab5_Dynamic::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(10, 100);
scatter.AddSeries(s1).Legend("U-t").Units("V", "s").NoMark();
scatter.AddSeries(s2).Legend("I-t").Units("A", "s").NoMark();
scatter.SetFastViewX(true).SetSequentialXAll(true);
bStart <<=(THISBACK(Start));
bStop <<=(THISBACK(Stop));
bReset <<=(THISBACK(Reset));
bPgDown <<=(THISBACK(PgDown));
bPgUp <<=(THISBACK(PgUp));
bPlus <<=(THISBACK(Plus));
bMinus <<=(THISBACK(Minus));
t = 0;
bStop.Disable();
}
void Tab5_Dynamic::Animate()
{
s1 << Pointf(t, 50+20*sin(t));
s2 << Pointf(t, 50+30*cos(t));
scatter.Refresh();
t += 0.1;
if((t-scatter.GetXMin()) >= scatter.GetXRange())
scatter.SetXYMin(scatter.GetXMin() + 0.1, 0);
}
void Tab5_Dynamic::Start()
{
SetTimeCallback(-5, THISBACK(Animate));
bStart.Disable();
bStop.Enable();
}
void Tab5_Dynamic::Stop()
{
KillTimeCallback();
bStart.Enable();
bStop.Disable();
}
void Tab5_Dynamic::Reset()
{
t = 0;
s1.Clear();
s2.Clear();
scatter.SetXYMin(0, 0);
scatter.Refresh();
}
void Tab5_Dynamic::PgDown()
{
scatter.SetXYMin(scatter.GetXMin()-5, 0);
scatter.Refresh();
}
void Tab5_Dynamic::PgUp()
{
scatter.SetXYMin(scatter.GetXMin()+5, 0);
scatter.Refresh();
}
void Tab5_Dynamic::Plus()
{
scatter.SetRange(scatter.GetXRange()/2, 100);
scatter.Refresh();
}
void Tab5_Dynamic::Minus()
{
scatter.SetRange(scatter.GetXRange()*2, 100);
scatter.Refresh();
}
ScatterDemo *Construct5()
{
static Tab5_Dynamic tab;
return &tab;
}
INITBLOCK {
RegisterExample("Dynamic", Construct5, __FILE__);
}
tab10_User.cpp
#include "ScatterCtrl_Demo.h"
#define IMAGECLASS Symbol
#define IMAGEFILE <ScatterCtrl_Demo/symbol.iml>
#include <Draw/iml.h>
class MyPlot : public SeriesPlot {
private:
template <class T>
void DoPaint(T& w, const double &scale, Vector<Pointf> &p, const double opacity,
double thick, const Color &color, String pattern, const Color &background,
const Color &fillColor, double y0) const {
Vector<Pointf> t;
t.SetCount(3);
for (int i = 0; i < p.GetCount(); ++i) {
t[0].x = p[i].x + 80;
t[0].y = y0;
t[1] = p[i];
t[2].x = p[i].x - 80;
t[2].y = y0;
if (!IsNull(fillColor))
FillPolylineOpa(w, t, scale, opacity, background, fillColor);
DrawPolylineOpa(w, t, scale, 1, thick, color, pattern, background);
}
}
public:
void Paint(Draw& w, Vector<Pointf> &p, const double &scale, const double opacity,
double thick, const Color &color, String pattern, const Color &background,
const Color &fillColor, double , double , double y0, double ,
bool ) const {
DoPaint(w, scale, p, opacity, fround(thick), color, pattern, background, fillColor, y0);
}
void Paint(Painter& w, Vector<Pointf> &p, const double &scale, const double opacity,
double thick, const Color &color, String pattern, const Color &background,
const Color &fillColor, double , double , double y0, double ,
bool ) const {
DoPaint(w, scale, p, opacity, fround(thick), color, pattern, background, fillColor, y0);
}
};
class MyMark : public MarkPlot {
private:
template <class T>
void DoPaint(T& w, const double& scale, const Point& cp, const double& , const Color& ) const {
Size bugSize = Symbol::bug().GetSize()*scale;
w.DrawImage(cp.x - bugSize.cx/2, cp.y - bugSize.cy/2, bugSize.cx, bugSize.cy, Symbol::bug());
}
public:
void Paint(Draw &p, const double& scale, const Point& cp, const double& size, const Color& markColor,
const double&, const Color&) const {
DoPaint(p, scale, cp, size, markColor);
}
void Paint(Painter &p, const double& scale, const Point& cp, const double& size, const Color& markColor,
const double&, const Color&) const {
DoPaint(p, scale, cp, size, markColor);
}
};
void Tab10_User::Init()
{
CtrlLayout(*this);
SizePos();
sy[0] = 18; sy[1] = 29; sy[2] = 23; sy[3] = 25; sy[4] = 20;
sx[0] = 10; sx[1] = 20; sx[2] = 30; sx[3] = 40; sx[4] = 50;
scatter.AddSeries(sx, sy, 5).Legend("Series").PlotStyle<MyPlot>().MarkStyle<MyMark>()
.Stroke(3, LtGreen()).Fill(LtGray());
scatter.SetRange(60, 50).SetMajorUnits(10, 10);
scatter.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg().Responsive();
}
ScatterDemo *Construct10()
{
static Tab10_User tab;
return &tab;
}
INITBLOCK {
RegisterExample("User graph", Construct10, __FILE__);
SeriesPlot::Register<MyPlot>("My plot");
MarkPlot::Register<MyMark>("My mark");
}
tab8_Secondary.cpp
#include "ScatterCtrl_Demo.h"
void Tab8_Secondary::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(13, 50, 20).SetMajorUnits(1, 10).SetXYMin(0,-20, 1000);
scatter.SetMouseHandling(true, true);
s3 <<Pointf(1,-12)<<Pointf(2,-17)<<Pointf(3,-13)<<Pointf(4,15)<<Pointf(5,18)<<Pointf(6,25)<<Pointf(7,27)<<Pointf(8,20)<<Pointf(9,5)<<Pointf(10,-10)<<Pointf(11,24)<<Pointf(12,17);
double barWidth = 0.4;
scatter.AddSeries(s3).Legend("Speed").Units("km/h").PlotStyle<BarSeriesPlot>().BarWidth(barWidth).NoMark().Stroke(2, Gray()).Dash(LINE_SOLID).Fill(LtGray());
s1<<Pointf(1,-6)<<Pointf(2,-4)<<Pointf(3,2)<<Pointf(4,8)<<Pointf(5,8)<<Pointf(6,15)<<Pointf(7,20)<<Pointf(8,30)<<Pointf(9,14)<<Pointf(10,10)<<Pointf(11,3)<<Pointf(12,-11);
scatter.AddSeries(s1).Legend("Temperature").Units("ºC").Stroke(3, Null).Dash(LINE_DASHED).MarkStyle<SquareMarkPlot>();
s2 <<Pointf(1,1008)<<Pointf(2,1012)<<Pointf(3,1016)<<Pointf(4,1012)<<Pointf(5,1008)<<Pointf(6,1016)<<Pointf(7,1012)<<Pointf(8,1004)<<Pointf(9,1000)<<Pointf(10,1001)<<Pointf(11,1000)<<Pointf(12,1002);
scatter.AddSeries(s2).Legend("Pressure").Units("mBar").Stroke(3, Null).Dash("15 6 6 6 3 6 6 6").SetDataSecondaryY();
scatter.SetDrawY2Reticle();
scatter.cbModifFormatX = THISBACK(MyFormat);
scatter.cbModifFormatDeltaX = THISBACK(MyFormatDelta);
scatter.SetMaxRange(40).SetMinRange(2, 20)
.SetLabelY("Temperature")
.SetLabelY2("Pressure")
.SetMarkWidth(1, 4);
scatter.ShowInfo()
.ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
}
ScatterDemo *Construct8()
{
static Tab8_Secondary tab;
return &tab;
}
INITBLOCK {
RegisterExample("Secondary Y", Construct8, __FILE__);
}
tab19_Surf.cpp
#include "ScatterCtrl_Demo.h"
void Tab19_Surf::Init()
{
CtrlLayout(*this);
SizePos();
numColor <<= 4;
numColor <<= THISBACK(OnChange);
opContinuous <<= true;
opContinuous <<= THISBACK(OnChange);
dataType = 0;;
dataType <<= THISBACK(OnChange);
rainbow.Add(BLUE_YELLOW_RED, "BLUE_YELLOW_RED").Add(RED_YELLOW_BLUE, "RED_YELLOW_BLUE")
.Add(GREEN_YELLOW_RED, "GREEN_YELLOW_RED").Add(RED_YELLOW_GREEN, "RED_YELLOW_GREEN")
.Add(WHITE_BLACK, "WHITE_BLACK").Add(BLACK_WHITE, "BLACK_WHITE");
rainbow = scatter.SurfRainbow();
rainbow << THISBACK(OnChange);
interpolation.Add(TableInterpolate::NO, "NO").Add(TableInterpolate::BILINEAR, "BILINEAR");
interpolation = TableInterpolate::BILINEAR;
interpolation << THISBACK(OnChange);
zoom = true;
zoom << THISBACK(OnChange);
scatter.ShowInfo().ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg()
.SetMouseHandling(true, true).Responsive(true)
.SetRainbowPaletteSize(Size(20, 200))
.SetRainbowPaletteAnchor(ScatterDraw::LEGEND_POS::LEFT_TOP)
.SetRainbowPalettePos(Point(20, 20))
.SetRainbowPaletteFont(StdFont().Height(10))
.SurfUnits("kg").SurfUnitsPos(ScatterDraw::UNITS_LEFT)
.SurfLegendPos(ScatterDraw::LEGEND_RIGHT);
for (double x = 0; x <= 5; x += 1)
xAxis << x;
yAxis << 0 << 4 << 5 << 6 << 7 << 8 << 9 << 10 << 11 << 12 << 13 << 14 << 15;
zData << 0 << 0 << 0 << 0 << 0 << 0
<< 0 << 402 << 2501 << 2099 << 48231 << 89315
<< 0 << 1835 << 9889 << 36124 << 78603 << 101067
<< 0 << 4627 << 24211 << 61689 << 117905 << 151600
<< 0 << 7170 << 37887 << 96988 << 185785 << 239028
<< 0 << 7144 << 39134 << 106627 << 213241 << 277208
<< 0 << 6290 << 33302 << 95397 << 196494 << 256218
<< 0 << 6931 << 32175 << 84656 << 165247 << 211670
<< 0 << 6117 << 27070 << 68657 << 130224 << 165038
<< 0 << 3849 << 17985 << 47400 << 91425 << 116321
<< 0 << 2390 << 12142 << 33214 << 65323 << 83722
<< 0 << 1741 << 9541 << 26098 << 51917 << 67241
<< 0 << 1337 << 7783 << 21287 << 42703 << 55722;
data.Init(zData, xAxis, yAxis, TableInterpolate::BILINEAR, false);
Vector<double> vals;
for (double val = 50000; val <= 250000; val += 50000)
vals << val;
isolines = data.GetIsolines(vals, Rectf(0, 16, 5, 0), 0.1, 0.1);
Vector<Pointf> numbersWhere;
numbersWhere << Pointf(4.7, 8) << Pointf(1, 14);
numbersPos = Intersection(numbersWhere, isolines);
for (int i = 0; i < vals.GetCount(); ++i)
labels << Format("%.0f kg", vals[i]);
for (double x = -10; x <= 10; x += 1)
xAxisFun << x;
for (double y = -10; y <= 10; y += 1)
yAxisFun << y;
zDataFun.SetCount((xAxisFun.GetCount()-1)*(yAxisFun.GetCount()-1));
for (int iy = 0; iy < yAxisFun.GetCount()-1; iy++) {
double y = (yAxisFun[iy] + yAxisFun[iy+1])/2.;
for (int ix = 0; ix < xAxisFun.GetCount()-1; ix++) {
double x = (xAxisFun[ix] + xAxisFun[ix+1])/2.;
zDataFun[ix + iy*(xAxisFun.GetCount() - 1)] = sin(sqrt(x*x + y*y + 64))/sqrt(x*x + y*y + 64);
}
}
dataFun.Init(zDataFun, xAxisFun, yAxisFun, TableInterpolate::BILINEAR, true);
funData.Init([=](double x, double y) {return sin(sqrt(x*x + y*y + 64))/sqrt(x*x + y*y + 64);}, -10, 10, -10, 10);
OnChange();
}
void Tab19_Surf::OnChange() {
labelInterpolation.Enable(dataType < 2);
interpolation.Enable(dataType < 2);
scatter.SurfNumColor(~numColor, ~opContinuous).SurfRainbow((RAINBOW)int(rainbow.GetKey(rainbow.GetIndex())));
if (dataType == 0)
Init_DatasetSimple();
else if (dataType == 1)
Init_Dataset();
else if (dataType == 2)
Init_DataExplicit();
else
scatter.RemoveSurf();
if (zoom)
scatter.ZoomToFit(true, true);
scatter.Refresh();
}
void Tab19_Surf::Init_DatasetSimple() {
scatter.RemoveAllSeries();
scatter.AddSeries(isolines).Legend("Isoline").Stroke(0.5, White).ShowSeriesLegend(false).NoMark();
scatter.AddSeries(numbersPos).Stroke(0).AddLabelSeries(labels, 0, 0, StdFont().Bold(), ALIGN_CENTER, White())
.ShowSeriesLegend(false).NoMark();
data.Inter((TableInterpolate::Interpolate)int(interpolation.GetKey(interpolation.GetIndex())));
scatter.AddSurf(data)
.SetRainbowPaletteTextColor(White)
.SetSurfMinZ(0).SetSurfMaxZ(300000);
}
void Tab19_Surf::Init_Dataset() {
scatter.RemoveAllSeries();
dataFun.Inter((TableInterpolate::Interpolate)int(interpolation.GetKey(interpolation.GetIndex())));
scatter.AddSurf(dataFun);
scatter.SetRainbowPaletteTextColor(Black);
scatter.ZoomToFitZ();
}
void Tab19_Surf::Init_DataExplicit() {
scatter.RemoveAllSeries();
scatter.AddSurf(funData);
scatter.SetRainbowPaletteTextColor(Black);
scatter.ZoomToFitZ();
}
ScatterDemo *Construct19()
{
static Tab19_Surf tab;
return &tab;
}
INITBLOCK {
RegisterExample("2D Surface", Construct19, __FILE__);
}
tab4_Formatting.cpp
#include "ScatterCtrl_Demo.h"
void Tab4_Formatting::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(13, 50)
.SetMajorUnits(1, 10)
.SetXYMin(0, -20);
scatter.SetMouseHandling(true).ShowContextMenu();
s1 <<Pointf(1,-6)<<Pointf(2,-4)<<Pointf(3,2)<<Pointf(4,8)<<Pointf(5,8)<<Pointf(6,15)<<Pointf(7,20)<<Pointf(8,25)<<Pointf(9,14)<<Pointf(10,10)<<Pointf(11,3)<<Pointf(12,-11);
scatter.AddSeries(s1).Legend("2005").Units("ºC");
s2 <<Pointf(1,-12)<<Pointf(2,-7)<<Pointf(3,0)<<Pointf(4,2)<<Pointf(5,10)<<Pointf(6,18)<<Pointf(7,25)<<Pointf(8,26)<<Pointf(9,15)<<Pointf(10,12)<<Pointf(11,7)<<Pointf(12,-6);
scatter.AddSeries(s2).Legend("2006").Units("ºC").MarkStyle<CircleMarkPlot>();
scatter.Graduation_FormatX(ScatterCtrl::MON)
.SetLabelY("Temperature")
.SetMarkWidth(1, 4)
.SetMinXmin(0).SetMaxXmax(13);
}
ScatterDemo *Construct4()
{
static Tab4_Formatting tab;
return &tab;
}
INITBLOCK {
RegisterExample("Formatting", Construct4, __FILE__);
}
tab9_Big.cpp
#include "ScatterCtrl_Demo.h"
void Tab9_Big::Init()
{
CtrlLayout(*this);
SizePos();
int dataRange = 100000;
scatter.SetRange(dataRange, 40).SetXYMin(0, -20);
scatter.SetMouseHandling(true).SetMaxRange(500000).SetMinRange(2);
for (int t = 0; t < dataRange; ++t) {
s1 << Pointf(t,20*sin(2*M_PI*t/dataRange));
s2 << Pointf(t,15*cos(2*M_PI*t/dataRange));
}
scatter.AddSeries(s1).Legend("series1").NoMark();
scatter.AddSeries(s2).Legend("series2").NoMark();
scatter.ShowAllMenus();
fastView.WhenAction = THISBACK(OnFastView);
sequentialX.WhenAction = THISBACK(OnSequentialX);
fastView = true;
sequentialX = true;
OnFastView();
OnSequentialX();
}
ScatterDemo *Construct9()
{
static Tab9_Big tab;
return &tab;
}
INITBLOCK {
RegisterExample("Big dataset", Construct9, __FILE__);
}
tab20_Pie.cpp
#include "ScatterCtrl_Demo.h"
void TabPie::Init()
{
CtrlLayout(*this);
chart.ShowPercent();
chart.AddCategory("Sunny", 8, ::Color(90, 150, 255));
chart.AddCategory("Cloudy", 4, ::Color(90, 255, 150));
chart.AddCategory("Rainy", 2, ::Color(250, 150, 90));
}
tab15_RangePlot.cpp
#include "ScatterCtrl_Demo.h"
void TabRangePlot::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetMouseHandling(true, true).ShowContextMenu().ShowPropertiesDlg().ShowProcessDlg();
for (double time = 0; time <= 10; time += 2.) {
Vector<double> &data = s1.Add();
double size = time * 10.;
data << time << ((size - 10) + Random(50)) << ((size - 4) + Random(50)) <<
((size + 4) + Random(50)) << ((size + 10) + Random(50));
}
idsRange << 1 << 2 << 3 << 4;
static Vector<int> idVoid;
scatter.AddSeries(s1, 0, Null, idVoid, idsRange, idVoid).Legend("Size").Units("m")
.MarkStyle<RangePlot>(RangePlot::MIN_AVG_MAX);
sdata << Pointf(0, 10) << Pointf(1, 14) << Pointf(2, 20) << Pointf(3, 30) << Pointf(4, 23) << Pointf(5, 25)
<< Pointf(6, 3) << Pointf(7, 21) << Pointf(8, 37) << Pointf(9, 32) << Pointf(10, 28);
for (int i = 0; i < sdata.GetCount(); ++i) {
smax << Pointf(sdata[i].x, sdata[i].y + 5 + Random(10));
smin << Pointf(sdata[i].x, sdata[i].y - 5 - Random(10));
}
dmax.Init(smax);
dmin.Init(smin);
range.Init(dmax, dmin);
scatter.AddSeries(range).Legend("Range").Closed(true).Stroke(0).Fill(LtCyan()).Opacity(0.5).NoMark();
scatter.AddSeries(smax).Legend("Max").Stroke(1, Blue()).NoMark();
scatter.AddSeries(smin).Legend("Min").Stroke(1, Blue()).NoMark();
scatter.AddSeries(sdata).Legend("Data").Stroke(4, Blue()).NoMark();
scatter.SetLabelY("Size").SetLabelX("time [seg]");
scatter.ShowButtons();
scatter.ZoomToFit(true, true, 0.2);
}
ScatterDemo *Construct15()
{
static TabRangePlot tab;
return &tab;
}
INITBLOCK {
RegisterExample("RangePlot", Construct15, __FILE__);
}
tabPie.cpp
#include "ScatterCtrl_Demo.h"
void TabPie::Init()
{
CtrlLayout(*this);
chart.ShowPercent();
chart.AddCategory("Sunny", 8, ::Color(90, 150, 255));
chart.AddCategory("Cloudy", 4, ::Color(90, 255, 150));
chart.AddCategory("Rainy", 2, ::Color(250, 150, 90));
}
tab3_ParametricFunctions.cpp
#include "ScatterCtrl_Demo.h"
Pointf opara1(double t) {return Pointf(0.5*cos(2*M_PI*t), 0.5*sin(2*M_PI*t));}
void para1(Pointf& xy, double t) {xy = Pointf(cos(2*M_PI*t), sin(2*M_PI*t));}
void para2(Pointf& xy, double t) {xy = Pointf(0.5*cos(6*M_PI*t)+3*t-1, 1.5+0.5*sin(6*M_PI*t));}
void para3(Pointf& xy, double t) {xy = Pointf(3+(0.5+t)*cos(6*M_PI*t), (0.5+t)*sin(6*M_PI*t));}
void para4(Pointf& xy, double t) {xy = Pointf(0, -0.25+0.5*t);}
void para1p(Pointf& xy, double t, double a, double b) {xy = Pointf(a*cos(2*M_PI*t),b*sin(2*M_PI*t));}
void para3p(Pointf& xy, double t, double a) {xy = Pointf(a+(0.5+t)*cos(6*M_PI*t),(0.5+t)*sin(6*M_PI*t));}
void Tab3_ParametricFunctions::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(7, 4);
scatter.SetMajorUnits(0.5, 0.5);
scatter.SetXYMin(-2, -2);
scatter.AddSeries(&opara1, 20).Legend("Circle 1");
scatter.AddSeries(¶1, 100).Legend("Circle 2").NoMark();
scatter.AddSeries(¶2, 100).Legend("Coil").NoMark();
scatter.AddSeries(¶3, 150).Legend("Spiral 1").NoMark();
scatter.AddSeries(STDBACK1(para3p, 2.5), 150, 0, 1).Legend("Spiral 2").NoMark();
scatter.AddSeries(¶4, 2).Legend("VLine").NoMark();
scatter.AddSeries([=](Pointf &xy, double t) {xy = Pointf(-0.25+0.5*t, 0);}, 2).Legend("HLine").NoMark();
scatter.AddSeries(STDBACK2(para1p, 4.0, 0.25), 50, 0, 1).Legend("Ellipse 1").NoMark();
scatter.AddSeries(STDBACK2(para1p, 2.0, 0.5), 50, 0, 1).Legend("Ellipse 2").NoMark();
}
ScatterDemo *Construct3()
{
static Tab3_ParametricFunctions tab;
return &tab;
}
INITBLOCK {
RegisterExample("Parametric Functions", Construct3, __FILE__);
}
tab6_Logarithmic.cpp
#include "ScatterCtrl_Demo.h"
void Tab6_Logarithmic::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(6,100);
scatter.SetMajorUnits(1,20);
scatter.ShowInfo();
scatter.SetMouseHandling(false, false);
s1 <<Pointf(log10(10.0),14)<<Pointf(log10(1e2),25)<<Pointf(log10(1e3),39)<<Pointf(log10(1e4),44)<<Pointf(log10(1e5),76);
scatter.AddSeries(s1).Legend("series1");
scatter.cbModifFormatX = THISBACK(FormatX);
scatter.cbModifFormatXGridUnits = THISBACK(FormatXGridUnits);
scatter.SetGridLinesX = THISBACK(SetGridLinesX);
}
void Tab6_Logarithmic::FormatX(String& s, int , double d)
{
double val = pow(10, d);
if (val >= 1 && val <= 1e5)
s = FormatDoubleExp(val, 0);
}
void Tab6_Logarithmic::FormatXGridUnits(String& s, int , double d)
{
double val = pow(10, d);
int valint = fround(val);
if (abs(val - double(valint)) < 0.0001) {
while (valint < -9 || valint > 9)
valint /= 10;
if (valint == 1)
s = FormatDoubleExp(pow(10, d), 0);
}
}
void Tab6_Logarithmic::SetGridLinesX(Vector<double>& unitsX)
{
for(int i = 0; scatter.GetXMinUnit() + i*scatter.GetMajorUnitsX() <= scatter.GetXRange(); i++) {
for (int ii = 0; ii < 9; ++ii)
unitsX << scatter.GetXMinUnit() + i*scatter.GetMajorUnitsX() + (log10((ii+1)*10)-1)*scatter.GetMajorUnitsX();
}
}
ScatterDemo *Construct6()
{
static Tab6_Logarithmic tab;
return &tab;
}
INITBLOCK {
RegisterExample("Log", Construct6, __FILE__);
}
tab7_Operations.cpp
#include "ScatterCtrl_Demo.h"
void Tab7_Operations::Init()
{
CtrlLayout(*this);
SizePos();
scatter.SetRange(80, 100);
bAddSeries <<= THISBACK(AddSeries);
bRemoveLastSeries <<= THISBACK(RemoveLastSeries);
bRemoveFirstSeries <<= THISBACK(RemoveFirstSeries);
bRemoveAllSeries <<= THISBACK(RemoveAllSeries);
}
void Tab7_Operations::AddSeries()
{
int num = scatter.GetCount();
double f = 1 + num*1.1;
Vector<Pointf> &s = series.Add();
s << Pointf(10, 3*f) << Pointf(30, 7*f) << Pointf(50, 12*f) << Pointf(70, 10*f);
scatter.AddSeries(s).Legend(String("series") + AsString(num));
}
void Tab7_Operations::RemoveFirstSeries()
{
if (scatter.IsEmpty())
return;
scatter.RemoveSeries(0);
series.Remove(0);
}
void Tab7_Operations::RemoveLastSeries()
{
if (scatter.IsEmpty())
return;
int topIndex = scatter.GetCount()-1;
scatter.RemoveSeries(topIndex);
series.Remove(topIndex);
}
void Tab7_Operations::RemoveAllSeries()
{
scatter.RemoveAllSeries();
series.Clear();
}
ScatterDemo *Construct7()
{
static Tab7_Operations tab;
return &tab;
}
INITBLOCK {
RegisterExample("Operations", Construct7, __FILE__);
}
tab14.cpp
#include "ScatterCtrl_Demo.h"
void TabUserEquation::Init()
{
CtrlLayout(*this);
SizePos();
equation <<= "25 + 10*sin(0.5*x + 5)";
fromX <<= 0;
toX <<= 100;
fromY <<= 0;
toY <<= 50;
update.WhenAction = THISBACK(OnUpdate);
scatter.SetMouseHandling(true, true);
OnUpdate();
}
void TabUserEquation::OnUpdate()
{
if (fromX >= toX || fromY >= toY) {
Exclamation("Wrong limits");
return;
}
userEquation.Init("User equation", equation);
scatter.RemoveAllSeries();
scatter.AddSeries(userEquation).Legend(userEquation.GetFullName()).NoMark().Stroke(2);
scatter.SetXYMin(fromX, fromY);
scatter.SetRange(toX - fromX, toY - fromY);
scatter.FitToData(true);
}
ScatterDemo *ConstructUserEquation()
{
static TabUserEquation tab;
return &tab;
}
INITBLOCK {
RegisterExample("User equation", ConstructUserEquation, __FILE__);
}
tab11_Trend.cpp
#include "ScatterCtrl_Demo.h"
void Tab11_Trend::Init()
{
CtrlLayout(*this);
SizePos();
seriesList.Add("Series 1").Add("Series 2");
seriesList = "Series 1";
seriesList.WhenAction = THISBACK(OnSeries);
scatter.SetMouseHandling(true, true).ShowContextMenu().ShowPropertiesDlg()
.ShowProcessDlg().Responsive();
grid.AddColumn("Type", 10);
grid.AddColumn("Equation", 40);
grid.AddColumn("R2", 5);
OnSeries();
}
void Tab11_Trend::OnSeries() {
double l_r2, p2_r2, p4_r2, f_r2, exp_r2, rat1_r2, s_r2;
scatter.RemoveAllSeries();
grid.Clear();
if (seriesList == "Series 1") {
scatter.SetRange(5, 1500).SetMajorUnits(1, 250).SetXYMin(-3, 0);
s1x.Clear();
s1y.Clear();
s1x << -3.067E0 << -2.981E0 << -2.921E0 << -2.912E0 << -2.840E0 << -2.797E0 << -2.702E0 << -2.699E0 << -2.633E0 << -2.481E0 << -2.363E0 << -2.322E0 << -1.501E0 << -1.460E0 << -1.274E0 << -1.212E0 << -1.100E0 << -1.046E0 << -0.915E0 << -0.714E0 << -0.566E0 << -0.545E0 << -0.400E0 << -0.309E0 << -0.109E0 << -0.103E0 << 0.010E0 << 0.119E0 << 0.377E0 << 0.790E0 << 0.963E0 << 1.006E0 << 1.115E0 << 1.572E0 << 1.841E0 << 2.047E0 << 2.200E0;
s1y << 80.574E0 << 84.248E0 << 87.264E0 << 87.195E0 << 89.076E0 << 89.608E0 << 89.868E0 << 90.101E0 << 92.405E0 << 95.854E0 << 100.696E0 << 101.060E0 << 401.672E0 << 390.724E0 << 567.534E0 << 635.316E0 << 733.054E0 << 759.087E0 << 894.206E0 << 990.785E0 << 1090.109E0 << 1080.914E0 << 1122.643E0 << 1178.351E0 << 1260.531E0 << 1273.514E0 << 1288.339E0 << 1327.543E0 << 1353.863E0 << 1414.509E0 << 1425.208E0 << 1421.384E0 << 1442.962E0 << 1464.350E0 << 1468.705E0 << 1447.894E0 << 1457.628E0;
VectorXY vs1(s1x, s1y);
linear.Fit(vs1, l_r2);
poly2.SetDegree(2);
poly2.Fit(vs1, p2_r2);
poly4.SetDegree(4);
poly4.Fit(vs1, p4_r2);
fourier.SetDegree(3);
fourier.Fit(vs1, f_r2);
exponential.Fit(vs1, exp_r2);
rational1.Fit(vs1, rat1_r2);
sin.GuessCoeff(vs1);
sin.Fit(vs1, s_r2);
scatter.AddSeries(s1x, s1y).Legend("Series 1").MarkStyle<RhombMarkPlot>().MarkWidth(10).NoPlot();
} else {
s2x.Clear();
s2y.Clear();
s2y << -0.9 << -1.1 << 2.8 << -3.4 << 2.7 << -0.9 << -1.1 << 2.8 << -3.4 << 2.7 << -0.9 << -1.1 << 2.8 << -3.4 << 2.7 << -0.7 << -1.2;
s2x << 0 << 0.6 << 1.2 << 1.8 << 2.5 << 3.1 << 3.7 << 4.3 << 5.0 << 5.6 << 6.2 << 6.9 << 7.5 << 8.1 << 8.7 << 9.4 << 10.0;
VectorXY v2(s2x, s2y);
linear.Fit(v2, l_r2);
poly2.SetDegree(2);
poly2.Fit(v2, p2_r2);
poly4.SetDegree(4);
poly4.Fit(v2, p4_r2);
fourier.SetDegree(3);
fourier.Fit(v2, f_r2);
exponential.Fit(v2, exp_r2);
rational1.Fit(v2, rat1_r2);
sin.GuessCoeff(v2);
sin.Fit(v2, s_r2);
scatter.AddSeries(s2x, s2y).Legend("Series 2").MarkStyle<RhombMarkPlot>().MarkWidth(10).NoPlot();
}
if (l_r2 > 0.5)
scatter.AddSeries(linear).Legend(linear.GetFullName()).NoMark().Stroke(2);
if (p2_r2 > 0.5)
scatter.AddSeries(poly2).Legend(poly2.GetFullName()).NoMark().Stroke(2);
if (p4_r2 > 0.5)
scatter.AddSeries(poly4).Legend(poly4.GetFullName()).NoMark().Stroke(2);
if (f_r2 > 0.5)
scatter.AddSeries(fourier).Legend(fourier.GetFullName()).NoMark().Stroke(2);
if (exp_r2 > 0.5)
scatter.AddSeries(exponential).Legend(exponential.GetFullName()).NoMark().Stroke(2);
if (rat1_r2 > 0.5)
scatter.AddSeries(rational1).Legend(rational1.GetFullName()).NoMark().Stroke(2);
if (s_r2 > 0.5)
scatter.AddSeries(sin).Legend(sin.GetFullName()).NoMark().Stroke(2);
scatter.ZoomToFit(true, true);
scatter.Refresh();
grid.Add(linear.GetFullName(), linear.GetEquation(), FormatDoubleFix(l_r2, 5));
grid.Add(poly2.GetFullName(), poly2.GetEquation(), FormatDoubleFix(p2_r2, 5));
grid.Add(poly4.GetFullName(), poly4.GetEquation(), FormatDoubleFix(p4_r2, 5));
grid.Add(fourier.GetFullName(), fourier.GetEquation(), FormatDoubleFix(f_r2, 5));
grid.Add(exponential.GetFullName(), exponential.GetEquation(), FormatDoubleFix(exp_r2, 5));
grid.Add(rational1.GetFullName(), rational1.GetEquation(), FormatDoubleFix(rat1_r2, 5));
grid.Add(sin.GetFullName(), sin.GetEquation(), FormatDoubleFix(s_r2, 5));
grid.SetSortColumn(2, true);
}
ScatterDemo *Construct11()
{
static Tab11_Trend tab;
return &tab;
}
INITBLOCK {
RegisterExample(t_("Trend Lines"), Construct11, __FILE__);
}
ScatterCtrl_Demo.lay
LAYOUT(ScatterCtrl_Demo, 624, 352)
ITEM(Button, bPreview, SetLabel(t_("Print Preview")).LeftPosZ(4, 88).BottomPosZ(4, 20))
ITEM(Button, bSavePNG, SetLabel(t_("Save as PNG")).LeftPosZ(4, 88).BottomPosZ(28, 20))
ITEM(Button, bSaveJPG, SetLabel(t_("Save as JPEG")).LeftPosZ(96, 80).BottomPosZ(28, 20))
ITEM(Button, bSaveEMF, SetLabel(t_("Save as EMF")).LeftPosZ(108, 68).BottomPosZ(52, 20))
ITEM(Button, bCopyClipboard, SetLabel(t_("Copy to Clipboard")).LeftPosZ(4, 100).BottomPosZ(52, 20))
ITEM(DropList, paintMode, LeftPosZ(4, 172).BottomPosZ(77, 19))
ITEM(Label, dv___6, SetLabel(t_("Painting Mode")).LeftPosZ(4, 172).BottomPosZ(97, 19))
ITEM(ArrayCtrl, examplesList, LeftPosZ(4, 168).VSizePosZ(28, 124))
ITEM(Label, dv___8, SetLabel(t_("Choose example")).LeftPosZ(4, 168).TopPosZ(4, 21))
END_LAYOUT
LAYOUT(Tab1Basic, 428, 296)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Basic Test")).SetPlotAreaRightMargin(20).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 4))
END_LAYOUT
LAYOUT(Tab2Functions, 380, 292)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Functions")).SetPlotAreaLeftMargin(40).SetPlotAreaRightMargin(20).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetGridWidth(2).SetLegendAnchor(2).SetLegendPosX(20).SetLegendPosY(20).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(16, 12).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(Tab3ParametricFunctions, 384, 284)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Parametric functions demo")).SetTitleColor(White).SetPlotAreaTopMargin(20).SetPlotAreaColor(Color(229, 229, 229)).SetLabelsColor(White).SetAxisColor(Color(28, 212, 0)).SetGridColor(Color(28, 127, 0)).SetLegendAnchor(2).SetColor(Color(198, 212, 255)).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(16, 16).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(Tab4Formatting, 400, 316)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Temperature Average. X Axis mouse zoom + scroll")).SetPlotAreaLeftMargin(60).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(40).SetLabelX(t_("Month")).SetLabelsFont(SansSerifZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(16, 16).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(Tab5Dynamic, 400, 288)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Dynamic")).SetPlotAreaLeftMargin(60).SetPlotAreaRightMargin(10).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(40).SetLabelX(t_("time")).SetLabelY(t_("Electric circuit performance")).SetLegendAnchor(2).SetLegendPosX(10).SetLegendPosY(10).SetTitleFont(StdFontZ(14).Bold()).HSizePosZ(16, 12).VSizePosZ(12, 36))
ITEM(Button, bStart, SetLabel(t_("Start")).LeftPosZ(16, 42).BottomPosZ(5, 23))
ITEM(Button, bStop, SetLabel(t_("Stop")).LeftPosZ(64, 42).BottomPosZ(5, 23))
ITEM(Button, bReset, SetLabel(t_("Reset")).LeftPosZ(112, 42).BottomPosZ(5, 23))
ITEM(Button, bPgDown, SetLabel(t_("<<")).RightPosZ(122, 30).BottomPosZ(5, 23))
ITEM(Button, bPgUp, SetLabel(t_(">>")).RightPosZ(86, 30).BottomPosZ(5, 23))
ITEM(Button, bPlus, SetLabel(t_("+")).RightPosZ(50, 30).BottomPosZ(5, 23))
ITEM(Button, bMinus, SetLabel(t_("-")).RightPosZ(14, 30).BottomPosZ(5, 23))
END_LAYOUT
LAYOUT(Tab6Logarithmic, 400, 280)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Logarithmic Scale")).SetPlotAreaTopMargin(20).SetLegendAnchor(2).SetTitleFont(StdFontZ(14).Bold()).HSizePosZ(12, 12).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(Tab7Operations, 388, 300)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Operations")).SetPlotAreaLeftMargin(45).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(35).SetLabelX(t_("[x]")).SetLabelY(t_("[y]")).SetLegendAnchor(2).SetTitleFont(MonospaceZ(16).Bold()).HSizePosZ(12, 12).VSizePosZ(12, 36))
ITEM(Button, bAddSeries, SetLabel(t_("AddSeries")).LeftPosZ(12, 60).BottomPosZ(4, 20))
ITEM(Button, bRemoveLastSeries, SetLabel(t_("RemoveLastSeries")).LeftPosZ(76, 104).BottomPosZ(4, 20))
ITEM(Button, bRemoveFirstSeries, SetLabel(t_("RemoveFirstSeries")).LeftPosZ(184, 100).BottomPosZ(4, 20))
ITEM(Button, bRemoveAllSeries, SetLabel(t_("RemoveAllSeries")).LeftPosZ(288, 88).BottomPosZ(4, 20))
END_LAYOUT
LAYOUT(Tab8Secondary, 400, 316)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Temperature & Pressure Averages. X-Y Axis mouse zoom + scroll")).SetPlotAreaLeftMargin(60).SetPlotAreaRightMargin(70).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(50).SetLabelX(t_("Month")).SetLabelY(t_("Legend Y")).SetLabelY2(t_("Legend Y2")).SetLabelsFont(SansSerifZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(15).Bold()).HSizePosZ(16, 16).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(Tab9Big, 380, 348)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Big dataset")).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(16, 12).VSizePosZ(16, 76))
ITEM(Option, sequentialX, SetLabel(t_("SetSequentialX")).LeftPosZ(112, 92).BottomPosZ(8, 16))
ITEM(Option, fastView, SetLabel(t_("SetFastView")).LeftPosZ(16, 84).BottomPosZ(8, 16))
ITEM(Label, dv___3, SetLabel(t_("It includes two series with 100000 points.\nTo work with Painter it requires SetFastView and SetSequentialX to be true. \nIf not the program could get stuck.")).HSizePosZ(16, 4).BottomPosZ(28, 44))
END_LAYOUT
LAYOUT(Tab10User, 380, 292)
ITEM(ScatterCtrl, scatter, SetTitle(t_("User graph")).SetPlotAreaLeftMargin(60).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(16, 12).VSizePosZ(16, 16))
END_LAYOUT
LAYOUT(TabUserEquation, 672, 276)
ITEM(ScatterCtrl, scatter, SetTitle(t_("User equation")).SetPlotAreaLeftMargin(60).SetPlotAreaRightMargin(20).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 28))
ITEM(Label, dv___1, SetLabel(t_("to")).RightPosZ(140, 16).BottomPosZ(3, 21))
ITEM(Label, dv___2, SetLabel(t_("from y =")).RightPosZ(204, 44).BottomPosZ(3, 21))
ITEM(Label, dv___3, SetLabel(t_("User equation y = f(x) =")).LeftPosZ(8, 116).BottomPosZ(3, 21))
ITEM(Label, dv___4, SetLabel(t_("to")).RightPosZ(312, 16).BottomPosZ(3, 21))
ITEM(Label, dv___5, SetLabel(t_("from x =")).RightPosZ(376, 44).BottomPosZ(3, 21))
ITEM(EditString, equation, HSizePosZ(124, 432).BottomPosZ(5, 19))
ITEM(EditDouble, fromX, RightPosZ(332, 44).BottomPosZ(5, 19))
ITEM(EditDouble, toX, RightPosZ(264, 44).BottomPosZ(5, 19))
ITEM(EditDouble, fromY, RightPosZ(160, 44).BottomPosZ(5, 19))
ITEM(EditDouble, toY, RightPosZ(92, 44).BottomPosZ(5, 19))
ITEM(Button, update, SetLabel(t_("Update")).RightPosZ(4, 80).BottomPosZ(4, 20))
END_LAYOUT
LAYOUT(Tab11Trend, 504, 468)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Trend Line demo")).SetPlotAreaLeftMargin(50).SetPlotAreaTopMargin(20).SetPlotAreaColor(Color(229, 229, 229)).SetLegendAnchor(4).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(8, 8).VSizePosZ(8, 108))
ITEM(ArrayCtrl, grid, HSizePosZ(112, 8).BottomPosZ(4, 100))
ITEM(Label, dv___2, SetLabel(t_("Data series:")).LeftPosZ(8, 64).BottomPosZ(55, 21))
ITEM(Label, dv___3, SetLabel(t_("Trend Lines Details:")).LeftPosZ(8, 100).BottomPosZ(83, 21))
ITEM(DropList, seriesList, LeftPosZ(8, 100).BottomPosZ(37, 19))
END_LAYOUT
LAYOUT(Tab12Linked, 456, 464)
ITEM(ScatterCtrl, scatter1, SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(1).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).TopPosZ(4, 152))
ITEM(ScatterCtrl, scatter2, SetPlotAreaTopMargin(0).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(1).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).TopPosZ(152, 152))
ITEM(ScatterCtrl, scatter3, SetPlotAreaTopMargin(0).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(1).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(300, 4))
ITEM(Option, link, SetLabel(t_("Link")).RightPosZ(4, 56).TopPosZ(4, 16))
END_LAYOUT
LAYOUT(TabRangePlot, 420, 236)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Range Plot")).SetPlotAreaLeftMargin(60).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(40).SetLabelsFont(StdFontZ(11)).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 4))
END_LAYOUT
LAYOUT(TabSurf, 468, 292)
ITEM(ScatterCtrl, scatter, SetTitle(t_("2D Surface")).SetPlotAreaLeftMargin(40).SetPlotAreaRightMargin(20).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(40).SetLabelsFont(StdFontZ(11)).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(132, 4).VSizePosZ(4, 4))
ITEM(LabelBox, dv___1, SetLabel(t_("Data source")).LeftPosZ(4, 124).TopPosZ(0, 76))
ITEM(Switch, dataType, SetLabel(t_("Data set simple\nData set\nExplicit equation\nNo surface")).LeftPosZ(12, 108).TopPosZ(16, 60))
ITEM(EditIntSpin, numColor, Min(0).NotNull(true).LeftPosZ(72, 56).TopPosZ(124, 19))
ITEM(Label, labelInterpolation, SetLabel(t_("Data set interpolation")).LeftPosZ(4, 120).TopPosZ(80, 19))
ITEM(Label, dv___5, SetLabel(t_("Num. color:")).LeftPosZ(4, 68).TopPosZ(124, 19))
ITEM(DropList, interpolation, LeftPosZ(4, 124).TopPosZ(100, 19))
ITEM(Label, dv___7, SetLabel(t_("Rainbow style")).LeftPosZ(4, 100).TopPosZ(168, 19))
ITEM(DropList, rainbow, LeftPosZ(4, 124).TopPosZ(188, 19))
ITEM(Option, zoom, SetLabel(t_("Zoom to fit")).LeftPosZ(4, 104).TopPosZ(212, 16))
ITEM(Option, opContinuous, SetLabel(t_("Continuous")).LeftPosZ(4, 88).TopPosZ(148, 16))
END_LAYOUT
LAYOUT(TabStackedPlot, 420, 244)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Stacked Plot")).SetPlotAreaLeftMargin(60).SetPlotAreaTopMargin(20).SetPlotAreaBottomMargin(40).SetLabelsFont(StdFontZ(11)).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 20))
ITEM(Switch, type, SetLabel(t_("Stacked\nStacked100")).LeftPosZ(4, 160).BottomPosZ(2, 16))
END_LAYOUT
LAYOUT(TabBubblePlot, 420, 236)
ITEM(ScatterCtrl, scatter, SetTitle(t_("Bubble Plot")).SetPlotAreaLeftMargin(80).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 4))
END_LAYOUT
LAYOUT(TabUserPaint, 428, 296)
ITEM(ScatterCtrl, scatter, SetTitle(t_("User paint")).SetPlotAreaLeftMargin(60).SetPlotAreaRightMargin(20).SetPlotAreaTopMargin(20).SetLabelsFont(StdFontZ(11)).SetLegendAnchor(2).SetTitleFont(SansSerifZ(14).Bold()).HSizePosZ(4, 4).VSizePosZ(4, 4))
END_LAYOUT
LAYOUT(TabPie, 536, 440)
ITEM(PieCtrl, chart, SetLegendBackColor(Color(229, 229, 229)).SetLegendLeft(20).SetTitle(t_("Average Weather")).SetFrame(ThinInsetFrame()).HSizePosZ(8, 12).VSizePosZ(40, 12))
ITEM(Label, dv___1, SetLabel(t_(" EXPERIMENTAL")).SetFrame(ThinInsetFrame()).LeftPosZ(8, 84).TopPosZ(8, 24))
END_LAYOUT
|