class TreeCtrl : public Ctrl
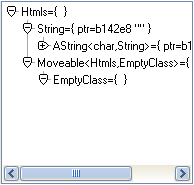
TreeCtrl hierarchy management is based on integer id numbers. Each node in tree (item) has associated integer id unique for the tree. Child nodes of item are organized as an array. TreeCtrl provides operation to insert child nodes at specified array position and to query child and parent nodes. Root item has fixed id 0.
Callback1<int> WhenOpen
Tree item was opened, parameter is the item id.
Callback1<int> WhenClose
Tree item was closed, parameter is the item id.
Callback WhenLeftClick
Tree item was clicked. Cursor identifies clicked item. You can get the click position within the item using GetItemClickPos method.
Callback WhenLeftDouble
Tree item was double-clicked. Cursor identifies clicked item. You can get the click position within the item using GetItemClickPos method.
Callback1<Bar&> WhenBar
Use to supply context menu.
Callback WhenSel
Cursor or selection has changed (including KillCursor).
Callback WhenDrag
Triggered when user attempts dragging an item.
Callback2<int, PasteClip&> WhenDropItem
This callback manages drag&drop into TreeCtrl items. The first parameter is an id of item. See PasteClip for more details.
Callback3<int, int, PasteClip&> WhenDropInsert
This callback manages drag&drop into insert positions between TreeCtrl items. The first parameter is an id of parent item, the second parameter is insert index within parent's child list. See PasteClip for more details.
Callback1<PasteClip&> WhenDrop
This callback manages drag&drop when WhenDropItem and WhenDropInsert do not apply - drop into empty area. See PasteClip for more details.
Callback WhenCursor
Cursor has changed (including KillCursor). Deprecated.
Callback WhenSelection
Selection has changed. Deprecated.
void SetRoot(const TreeCtrl::Node& n)
Sets the content of root item to n. Full deep copy of n is performed.
void SetRoot(const Image& img, Value v)
Sets image, key and value of root item. v is used both for key and value.
void SetRoot(const Image& img, Value key, Value text)
Sets the image, key and value of root item.
void SetRoot(const Image& img, Ctrl& ctrl, int cx = 0, int cy = 0)
Sets widget to be at root item. Only reference to ctrl is stored -> widget object lifetime must exceed TreeCtrl's lifetime. Dimensions of widget should be cx, cy. If either cx or cy are zero, GetMinSize dimension is used.
int Insert(int parentid, int i, const TreeCtrl::Node& n)
int Insert(int parentid, int i)
int Insert(int parentid, int i, const Image& img, Value value, bool withopen = false)
int Insert(int parentid, int i, const Image& img, Value key, Value value, bool withopen = false)
int Insert(int parentid, int i, const Image& img, Ctrl& c, int cx = 0, int cy = 0, bool wo = false)
int Insert(int parentid, int i, const Image& img, Value key, const String& value, bool withopen = false)
int Insert(int parentid, int i, const Image& img, Value key, const char *value, bool withopen = false)
Inserts child item to parent specified by id. Distinct variants set various attributes to TreeCtrl::Node of insterted item. Returns id of new item. Note: Last two overloads are to avoid overloading ambiguity.
int Add(int parentid, const TreeCtrl::Node& n)
int Add(int parentid)
int Add(int parentid, const Image& img, Value value, bool withopen = false)
int Add(int parentid, const Image& img, Value key, Value value, bool withopen = false)
int Add(int parentid, const Image& img, Ctrl& ctrl, int cx = 0, int cy = 0, bool withopen = false)
int Add(int parentid, const Image& img, Value key, const String& value, bool withopen = false)
int Add(int parentid, const Image& img, Value key, const char *value, bool withopen = false)
Inserts child item at the end of list of parent's child items. Parent is specified by id. Distinct variants set various attributes to TreeCtrl::Node of insterted item. Returns id of new item. Note: Last two overloads are to avoid overloading ambiguity.
void Remove(int id)
Removes item with id from the tree.
void RemoveChildren(int id)
Removes all child items from parent item with id.
void Swap(int id1, int id2)
Swaps two tree items.
void SwapChildren(int parentid, int i1, int i2)
Swaps parentid children with indices i1 and i2.
int GetChildCount(int id) const
Returns the number of child items of parent item with id.
int GetChild(int id, int i) const
Returns the identifier of child at index i of parent item with id.
int GetChildIndex(int parentid, int childid) const
Returns an index of child item of parentid with id childid.
int GetParent(int id) const
Returns the parent identifier of child with id.
Value Get(int id) const
Value GetValue(int id) const
Returns the value of item with id.
Value operator[](int id) const
Returns the key of item with id.
void Set(int id, Value v)
Sets the value and key of item with id.
void Set(int id, Value key, Value value)
Sets the value and key of item with id.
void SetValue(const Value& v)
Sets the value of item with cursor, key is unchanged.
void SetDisplay(int id, const Display& display)
Sets the display of id item.
void RefreshItem(int id)
Forces the repainting of item (e.g. when its appearance changes in a way that cannot be detected by TreeCtrl widget).
int GetLineCount()
Gets the current number of lines - visible items - in TreeCtrl.
int GetItemAtLine(int i)
Gets the id of item at line i.
int GetLineAtItem(int id)
Gets the line of item with id. If item is not visible, returns negative value.
Node GetNode(int id) const
Returns attributes of item with id.
void SetNode(int id, const TreeCtrl::Node& n)
Sets attributes.
bool IsValid(int id) const
Returns true if id represents a valid node id.
bool IsOpen(int id) const
Tests whether item is opened.
void Open(int id, bool open = true)
Opens or closes (if open is false) item with id - makes all child items visible.
void Close(int id)
Same as Open(id, false).
Vector<int> GetOpenIds() const
Returns all open node ids.
void OpenIds(const Vector<int>& ids)
Opens all valid ids.
void OpenDeep(int id, bool open = true)
Opens/closes item with id and all of its child items.
void CloseDeep(int id)
Same as OpenDeep(id, false).
void MakeVisible(int id)
Opens all parent items of item with id to make it visible.
void SetCursorLine(int i)
Sets cursor in the tree to be at visible item line i.
int GetCursorLine() const
Returns visible item line.
void KillCursor()
Removes cursor from tree.
void SetCursor(int id)
Sets cursor to item with id. If item is not visible, MakeVisible(id) is called first.
int GetCursor() const
Returns id of item that currently has cursor or negative value if there is none.
bool IsCursor() const
Returns true if there is cursor in the tree.
Point GetItemClickPos() const
Returns current mouse position within item Display rectangle when clicked.
Point GetScroll() const
Returns the scroll position of tree.
void ScrollTo(Point sc)
Scrolls tree back to value previously returned by GetScroll.
Value Get() const
Returns key of item with cursor or Null if there is none.
Value GetValue() const
Returns value of item with cursor or Null if there is none.
int Find(Value key)
Returns id of item with key or negative value if not found.
bool FindSetCursor(Value key)
Places cursor to first item with key, returns true if found.
void Sort(int id, const ValueOrder& order, bool byvalue = false)
Sorts child items of item with id using order sorting predicate. If byvalue is false, items are sorted by keys, if true, by values.
void SortDeep(int id, const ValueOrder& order, bool byvalue = false)
Sorts child items of item with id using order sorting predicate. If byvalue is false, items are sorted by keys, if true, by values. After sorting, it recursively calls SortDeep for all child items.
void Sort(int id, int (*compare)(const Value& v1, const Value& v2) = StdValueCompare, bool byvalue = false)
Sort with simplified predicate compare.
void SortDeep(int id, int (*compare)(const Value& v1, const Value& v2) = StdValueCompare, bool byvalue = false)
SortDeep with simplified predicate compare.
void SortByValue(int id, const ValueOrder& order)
void SortDeepByValue(int id, const ValueOrder& order)
void SortByValue(int id, int (*compare)(const Value& v1, const Value& v2) = StdValueCompare)
void SortDeepByValue(int id, int (*compare)(const Value& v1, const Value& v2) = StdValueCompare)
Convenience variants call their basic counterparts with byvalue equal to true.
void Sort(int id, const ValuePairOrder& order)
void Sort(int id, int (*compare)(const Value& k1, const Value& v1, const Value& k2, const Value& v2))
Sorts the children list of parent id. Note that if id is 0, the whole tree is sorted. The grandchildren are left intact.
void SortDeep(int id, const ValuePairOrder& order)
void SortDeep(int id, int (*compare)(const Value& k1, const Value& v1, const Value& k2, const Value& v2))
Sorts the subtree of parent id. Note that if id is 0, the whole tree is sorted. The grandchildren are sorted as well.
void Clear()
Removes all items from the tree.
void ClearSelection()
Clears any selection.
void SelectOne(int id, bool sel)
Selects/unselects single item with id.
bool IsSelected(int id) const
Returns true if item with id is selected.
bool IsSel(int id) const
Returns true if item with id is selected or has cursor.
int GetSelectCount() const
The number of selected items.
bool IsSelDeep(int id) const
True, if IsSel is true for the item or if IsSelDeep is true for its parent (if any item in parenthood chain is selected).
Vector<int> GetSel() const
Returns ids of all items with IsSel is true (note that it includes cursor item if there is no selection).
void Remove(const Vector<int>& id)
Removes a set of items, id is a list of item ids to remove.
void RemoveSelection()
Same as Remove(GetSel()) - removes all selected items.
void Dump()
Diagnostic dump of tree content to standard log. Exists in debug mode only.
TreeCtrl& NoCursor(bool b = true)
Tree does not allow cursor.
TreeCtrl& NoRoot(bool b = true)
Root item is not shown.
TreeCtrl& LevelCx(int cx)
Indentation per single hierarchy level. Default value is 16 pixels.
TreeCtrl& MultiSelect(bool b = true)
Allows selection of items.
TreeCtrl& NoBackground(bool b = true)
White background of tree is not painted.
In case that the display size of node value is greater than currently available area, attempts to show the complete value when cursor hovers over the node.
Same as PopUpEx(false).
TreeCtrl& MouseMoveCursor(bool m = true)
Mouse cursor moves tree cursor without clicking, just by moving the mouse over the tree.
TreeCtrl& Accel(bool a = true)
Activates simple keyboard accelerator. Node values are converted using StdFormat to text and then the first letter is used to find the appropriate item.
TreeCtrl& SetDisplay(const Display& d)
Sets the universal Display used for all node values. This can be overridden for particular node by assigning node specific Display.
TreeCtrl& HighlightCtrl(bool a = true)
Paints the background area of embedded widgets with the same color as that used to paint regular values - respects selection, cursor position etc.
TreeCtrl& RenderMultiRoot(bool a = true)
When active, no lines are drawn connecting zero level nodes (so it looks like there are multiple roots).
TreeCtrl& EmptyNodeIcon(const Image& a)
When RenderMultiRoot is active and there the node is zero level and has not elements, a is drawn on the left side to indicate it is empty.
TreeCtrl& SetScrollBarStyle(const ScrollBar::Style& s)
Assigns a chameleon visual style of scrollbar of TreeCtrl.
class Node
This class represents the content and appearance of single TreeCtrl node-item.
Node()
Default constructor.
Node(const Image& img, const Value& v)
Assigns icon, key and value.
Node(const Image& img, const Value& v, const Value& t)
Assigns icon, key and value.
Node(const Value& v)
Assigns key and value.
Node(const Value& v, const Value& t)
Assigns key and value.
Node(Ctrl& ctrl)
Assigns widget.
Node(const Image& img, Ctrl& ctrl, int cx = 0, int cy = 0)
Assigns icon, widget and its dimensions.
Image image
Node icon.
int margin
Space between icon and value of the item, or item's widget. Defaults to 2.
Value key
Item key. This is not displayed.
Value value
Item value.
const Display *display
Display used to render value. Defaults to NULL, which means StdDisplay is to be used.
Size size
Size of value area. Defaults to Null - in that case, value area size is determined by display->GetStdSize(value) or ctrl->GetMinSize() if widget for item is used.
Ptr<Ctrl> ctrl
Widget associated with item.
bool canopen
Item can be opened.
bool canselect
Item can be selected.
Node& SetImage(const Image& img)
Sets image.
Node& Set(const Value& v)
Sets both key and value to v.
Node& Set(const Value& v, const Value& t)
Sets key and value.
Node& SetDisplay(const Display& d)
Sets display.
Node& SetSize(Size sz)
Sets size.
Node& SetCtrl(Ctrl& _ctrl)
Sets widget.
Node& CanOpen(bool b = true)
Sets canopen flag.
Node& CanSelect(bool b)
Sets canselect flag.
|