1. Basics
To use a grid control in your application add the GridCtrl package to your project and then just add grid as any other control in layout editor (Complex-> GridCtrl) or manually put some code somewhere in your app constructor eg:
#include <CtrlLib/CtrlLib.h>
#include <GridCtrl/GridCtrl.h>
struct App : TopWindow
{
typedef App CLASSNAME;
GridCtrl grid;
App()
{
Add(grid.SizePos());
}
};
GUI_APP_MAIN
{
App().Run();
}
Now we have grid control spanned onto the main window. However grid without columns is useless. To add some columns write:
grid.AddColumn("Name");
grid.AddColumn("Age");
Let's add some data into it:
grid.Add("Ann", 21)
.Add("Jack", 34)
.Add("David", 15);
As you can see the first row of grid containing column names is painted differently. It is often called header (as in the array control), but here I call it fixed row (because there can me more than one fixed row). Next "lines" are just ordinary rows.
Once you've added data into the grid you can change it:
grid.Set(0, 0, "Daniel");
First argument of Set() is a row number, second is a column and the last - a new value to be set. Remember that the row 0 is the first row after fixed rows. To change the value of fixed item use SetFixed:
grid.SetFixed(0, 1, "Age of person");
If number of row/column in Set is greater than the number of total rows/columns - grid is automatically "stretched" to fit the new item1.
If you want to change value of current row you can omit the first argument of Set():
grid.Set(0, "Daniel 1");
In both cases you can use the short form:
grid(0, 0) = "Daniel";
grid(0) = "Daniel 1";
However there are two differences:
short form always updates internal data. That means if there is an edit control active above your item it's value won't change - only underlaying value will change.
short form never refreshes grid.
Short form are mainly dedicated to extremely fast updates and to use in callbacks (see next chapter)
Now let's do opposite. Let's get the data from grid. To do it simply use Get() method:
Value v0 = grid.Get(0, 0); // get value from row 0 and column 0
Value v1 = grid.Get(0); // get value from cursor row and column 0
Value v0 = grid(0, 0); // short form of case 1
Value v1 = grid(0); // short form of case 2
Get always returns a copy of internal data (short form returns reference to the internal value).
1) Not fully implemented in current version, only resizing of rows. Column resizing will be available in full 1.0 version.
2. Callbacks
The easiest method to add some interaction to the grid control is to use callbacks. There are many. The most basic are:
WhenLeftClick - called when left mouse button is pushed.
WhenLeftDouble - called when left mouse button is pushed twice.
WhenRowChange - called when cursor in grid changes its position.
WhenEnter - called when enter key is pressed.
Add to the code:
void ShowInfo()
{
PromptOK(Format("%s is %d years old", grid(0), grid(1)));
}
grid.WhenLeftDouble = THISBACK(ShowInfo);
After running the application and double clicking at first row you should see:
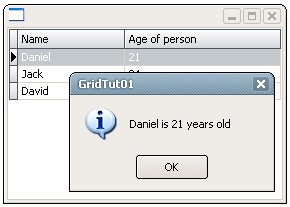
Now some funky stuff:
void RandomColor()
{
grid.GetRow().Bg(Color(rand() % 255, rand() % 255, rand() % 255));
}
grid.WhenRowChange = THISBACK(RandomColor);
Try to change cursor position (using cursor keys or mouse). After each position change the background color of the previous active row is changed.
3. Editing, indexes and integration with databases
Displaying static data is very useful, but in most cases some of it must be changed. One way is to show another window (eg as a reaction on double click) and put entered data from it into the grid. Second way is to edit data directly in grid. GridCtrl support two edit modes:
1. Row editing.
In this mode all edit controls binded to the columns are shown. You can move from one edit to another by pressing tab key (or enter if special switch is on).
2. Cell editing
In this mode only one edit control is displayed. Tab moves the cursor to the next edit control (if available).
Binding edits with columns is very easy. Let's make our example to allow edit name and age. First we need to declare edit controls:
EditString name;
EditInt age;
Then we simply call Edit method for each column:
grid.AddColumn("Name").Edit(name);
grid.AddColumn("Age").Edit(age);
Now you can press Enter or click double LMB to start editing. By default Tab skips from one cell to another (with binded edit). If it is the last editing cell pressing Tab adds a new row. There are several ways to change editing behaviour e.g:
grid.TabAddsRow(bool b) enables/disables adding new row after pressing tab key
grid.TabChangesRow(bool b) enables/disables changing row after pressing tab key
grid.EnterLikeTab(bool b) enables/disables emulation of tab by enter key
grid.OneClickEdit(bool b) enables/disables immediate editing after LMB click
4. Bulit-in toolbar, popup menu
5. Properties
6. Others
|