About Frames
Each U++ Ctrl (widget) has one or more frames. Frames are objects derived from CtrlFrame class that form appearance and functionality of area between outer Ctrl border and its view.
Frames are ordered and each has its index. Ctrl has always frame with index 0 and it is usually used to provide decorative border of Ctrl. If Ctrl does not need to have any decorative border, frame 0 can be set to NullFrame(). For example, StaticRect by default has NullFrame() as frame0:
TopWindow win;
StaticRect ctrl;
ctrl.Color(SLtCyan);
win.Add(ctrl.SizePos());
win.SetRect(0, 0, 100, 100);
win.Run();
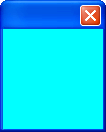
You can change frame 0 using SetFrame method:
ctrl.SetFrame(InsetFrame());
win.Run();
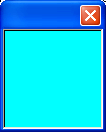
Note that CtrlFrame class interface is designed so that in case of specific Frames (like InsetFrame and other decorative static frames) one instance of frame object can be used for any number of Ctrl instances. In other cases, like ScrollBar and other frames containing frame subctrls, you need to use one Frame instance per single use.
Now we can add another decorative frame in our example:
ctrl.AddFrame(BlackFrame());
win.Run();
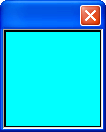
As you can see, frames add added from outer border to view area of Ctrl. To examine CtrlFrame class interface, let us create simple frame from scratch:
struct Frame1 : CtrlFrame {
virtual void FrameLayout(Rect& r) {
r.left += 20;
}
virtual void FrameAddSize(Size& sz) {
sz.cx += 20;
}
virtual void FramePaint(Draw& w, const Rect& r) {
w.DrawRect(r.left, r.top, 20, r.Height(), SLtGreen);
}
};
FrameLayout sets up internal layout of frame and decreases size of Ctrl rectangle. U++ starts from outer rectangle of Ctrl and calls FrameLayout for each frame. The resulting rectangle represents Ctrl's view. FrameAddSize represents opposite process - it is used to determine the required size of Ctrl to achieve the specified size of view, in other words, to compute the size of Ctrl from the size of its view with the current set of frames. Finally FramePaint allows painting in the frame area.
Now let us our example frame:
Frame1 frame1;
ctrl.InsertFrame(0, frame1);
win.Run();
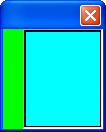
In more complex cases, where frame is to provide interactive behaviour - like e.g. ScrollBar or HeaderCtrl, it is needed to add some subctrls into the frame area. You can do this in FrameAdd and FrameRemove methods (FrameRemove being reserved for removing from frames) using SetFrameRect:
struct Frame2 : Frame1 {
Button x;
virtual void FrameLayout(Rect& r) {
x.SetFrameRect(r.left, r.top, 16, 16);
r.left += 16;
}
virtual void FrameAddSize(Size& sz) {
sz.cx += 16;
}
virtual void FramePaint(Draw& w, const Rect& r) {
w.DrawRect(r.left, r.top + 16, 20, r.Height() - 16, SLtMagenta);
}
virtual void FrameAdd(Ctrl& parent) {
parent.Add(x);
}
virtual void FrameRemove() {
x.Remove();
}
Frame2() {
x.LeftPos(0, 16).TopPos(0, 16).NoWantFocus();
}
};
.......
Frame2 frame2;
ctrl.AddFrame(frame2);
win.Run();
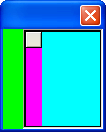
Many Ctrls are able to work both as regular view-area ctrls or as frames - it is achieved by deriving both from Ctrl and CtrlFrame. Examples are ScrollBar or HeaderCtrl.
You can also use utility templates FrameLeft, FrameRight, FrameTop and FrameBottom to add frame functionality to any existing Ctrl.
|