HelloWorld
Rudimentary HelloWorld application with menu and status bar
Description
Historically almost every program language or framework have some kind of "Hello world!" example and Ultimate++ framework is not exception:). So in this short tutorial I will try to explain you the basics of U++ framework with the help of HelloWorld U++ example.
So when you start HelloWorld application, and go to "File->About" you will see somethings like this:
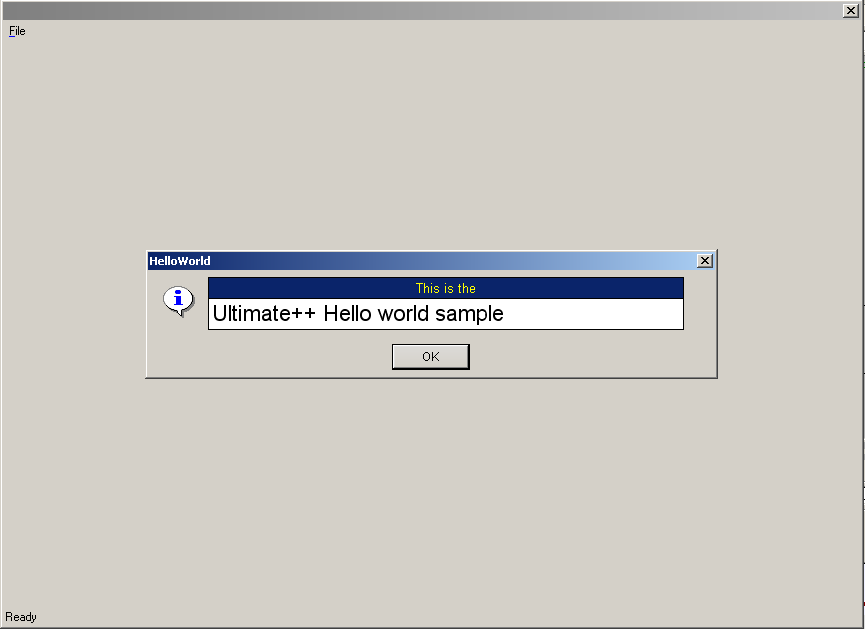
Let as see actual code for HelloWorld U++ application:
#include <CtrlLib/CtrlLib.h>
class HelloWorld : public TopWindow {
MenuBar menu;
StatusBar status;
void FileMenu(Bar& bar);
void About();
public:
typedef HelloWorld CLASSNAME;
HelloWorld();
};
void HelloWorld::About()
{
PromptOK("{{1@5 [@9= This is the]::@2 [A5@0 Ultimate`+`+ Hello world sample}}");
}
void HelloWorld::FileMenu(Bar& bar)
{
bar.Add("About..", THISBACK(About));
bar.Separator();
bar.Add("Exit", THISBACK(Close));
}
HelloWorld::HelloWorld()
{
AddFrame(menu);
AddFrame(status);
menu.Add("File", THISBACK(FileMenu));
status = "Welcome to the Ultimate++ !";
}
GUI_APP_MAIN
{
SetLanguage(LNG_ENGLISH);
HelloWorld().Run();
}
OK, lots of unknown stuff here, but lets go slowly line by line.
First of all we include "CtrlLib/CtrlLib.h"; this header includes most of the U++ widgets. By the way all U++ widgets have the base class Ctrl and that's way U++ widgets are also named "Ctrls".
Next we derived HelloWorld class from TopWindow which represent basic independent OS window with stuff like caption, close/zoom/restore buttons etc. In plain English it's our main window;)
Our HelloWorld window will have menu bar and status bar and in class they are saved like two private members.
MenuBar menu;
StatusBar status;
In constructor of HelloWorld with a help of AddFrame function we added menu bar and status bar. AddFrame will add those elements as Frames, placing them at border of TopWindow and reducing the view-area of window. Both MenuBar and StatusBar know where they should place self when used as Frames (MenuBar at the top, StatusBar at the bottom).
Next line in constructor is very interesting
menu.Add("File", THISBACK(FileMenu));
and deserves more explanation. U++ is using callbacks - callbacks can be described as a very generalized form of function pointers. Each Callback represents some kind of action (usually calling a certain function or a certain object method) that can be invoked at any time. Now,
THISBACK(x)
is a macro that expands to
callback(this, &CLASSNAME::x)
where x is name of method we want to call with this action. Function callback is a template function and in order to work you must define CLASSNAME variable and thats way every U++ class that use THISBACK macros has next line of code
typedef HelloWorld CLASSNAME;
So basically
menu.Add("File", THISBACK(FileMenu));
adds "File" menu in menu bar of our application and when a user click on "File" U++ will call corresponding FileMenu function from our HelloWorld class
void HelloWorld::FileMenu(Bar& bar)
{
bar.Add("About..", THISBACK(About));
bar.Separator();
bar.Add("Exit", THISBACK(Close));
}
What happens here is that when a user clicks on "File" menu, U++ creates a pop up menu bar and than calls FileMenu function which parameter is address of that created menu bar. Then FileMenu adds "About" and "Exit" choices, adds separator between them and binds actions to that choices to corresponding function calls from our HelloWorld object (see more detailed dicussion of callbacks involved here).
Last line of constructor is
status = "Welcome to the Ultimate++ !";
Class StatusBar has overloaded operator = which sets text of our status bar. Nice and clean.
The last function from HelloWorld class is
void HelloWorld::About()
{
PromptOK("{{1@5 [@9= This is the]::@2 [A5@0 Ultimate`+`+ Hello world sample}}");
}
Obviously when user click on "File"->"About" in menu this function is called. PromtOK is handy global function declared in "CtrlLib\RichText.h", and she pops up prompt dialog with nice information icon and OK button. Also this dialog has some information text displayed to user and this text is parameter of PromtOK function. Notice some strange characters in our info text, this are QTF codes and QTF is the native format for U++ rich texts (formatted texts).
And on the end we have
GUI_APP_MAIN
{
SetLanguage(LNG_ENGLISH);
HelloWorld().Run();
}
As you may guess, every program needs to have entry point where it execution begins, and in U++ this code begins with GUI_APP_MAIN macro. This macro is tacking care of initialization of U++ GUI application for appropriate OS platform, and on the end closing and exiting from framework.
Like every good framework U++ also have support for multi lingual programs, so first we set language of our application to English.
Then we make one object from HelloWorld class and call appropriate Run method defined in TopWindow class which starts our application.
Hello.cpp
#include <CtrlLib/CtrlLib.h>
using namespace Upp;
class HelloWorld : public TopWindow {
MenuBar menu;
StatusBar status;
public:
HelloWorld();
};
HelloWorld::HelloWorld()
{
Title("Hello world!");
AddFrame(menu);
AddFrame(status);
menu.Set([=](Bar& bar) {
menu.Sub("File", [=](Bar& bar) {
bar.Add("About..", [=] {
PromptOK("{{1@5 [@9= This is the]::@2 [A5@0 U`+`+ Hello world sample}}");
});
bar.Separator();
bar.Add("Exit", [=] { Close(); });
});
});
status = "Welcome to the U++!";
}
GUI_APP_MAIN
{
HelloWorld().Run();
}
|