SqlCtrls
This package demostrates the use of SqlCtrls GUI-SQL dialog mapping class
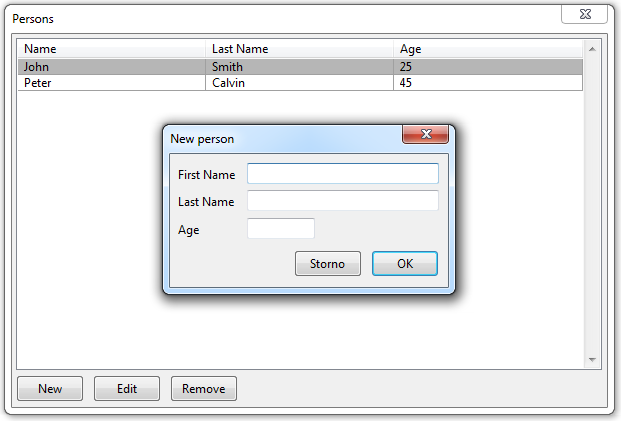
SqlCtrls.h
#ifndef _SqlCtrls_SqlCtrls_h
#define _SqlCtrls_SqlCtrls_h
#include <CtrlLib/CtrlLib.h>
#include <SqlCtrl/SqlCtrl.h>
#include <plugin/sqlite3/Sqlite3.h>
using namespace Upp;
#define LAYOUTFILE <SqlCtrls/SqlCtrls.lay>
#include <CtrlCore/lay.h>
#define SCHEMADIALECT <plugin/sqlite3/Sqlite3Schema.h>
#define MODEL <SqlCtrls/Model.sch>
#include "Sql/sch_header.h"
class PersonsDlg : public WithPersonsLayout<TopWindow> {
typedef PersonsDlg CLASSNAME;
void Create();
void Edit();
void Remove();
public:
PersonsDlg();
};
#endif
main.cpp
#include "SqlCtrls.h"
#ifdef _DEBUG
#include <Sql/sch_schema.h>
#endif
#include <Sql/sch_source.h>
struct PersonDlg : public WithPersonLayout<TopWindow> {
typedef PersonDlg CLASSNAME;
SqlCtrls ctrls;
PersonDlg();
};
PersonDlg::PersonDlg()
{
CtrlLayoutOKCancel(*this, "Person");
#ifdef flagSCH
ctrls(*this, PERSON); // matches widgets to columns based on Layout and schema introspection
#else
ctrls // "manual" variant
(NAME, name)
(LASTNAME, lastname)
(AGE, age)
;
#endif
}
void PersonsDlg::Create()
{
PersonDlg dlg;
dlg.Title("New person");
if(dlg.Execute() != IDOK)
return;
SQL * dlg.ctrls.Insert(PERSON);
int id = SQL.GetInsertedId();
list.ReQuery();
list.FindSetCursor(id);
}
void PersonsDlg::Edit()
{
int id = list.GetKey();
if(IsNull(id))
return;
PersonDlg dlg;
dlg.Title("Edit person");
if(!dlg.ctrls.Load(PERSON, ID == id))
return;
if(dlg.Execute() != IDOK)
return;
SQL * dlg.ctrls.Update(PERSON).Where(ID == id);
list.ReQuery();
list.FindSetCursor(id);
}
void PersonsDlg::Remove()
{
int id = list.GetKey();
if(IsNull(id) || !PromptYesNo("Delete row?"))
return;
SQL * SqlDelete(PERSON).Where(ID == id);
list.ReQuery();
}
PersonsDlg::PersonsDlg()
{
CtrlLayout(*this, "Persons");
create << THISFN(Create);
edit << THISFN(Edit);
remove << THISFN(Remove);
list.SetTable(PERSON);
list.AddKey(ID);
list.AddColumn(NAME, "Name");
list.AddColumn(LASTNAME, "Last Name");
list.AddColumn(AGE, "Age");
list.WhenLeftDouble = THISFN(Edit);
list.SetOrderBy(AGE, NAME);
list.Query();
}
GUI_APP_MAIN
{
Sqlite3Session sqlite3;
if(!sqlite3.Open(GetHomeDirFile("persons.db"))) {
LOG("Can't create or open database file\n");
return;
}
sqlite3.SetTrace();
SQL = sqlite3;
// Update the schema to match the schema described in "Model.sch"
#ifdef _DEBUG
SqlSchema sch(SQLITE3);
All_Tables(sch);
if(sch.ScriptChanged(SqlSchema::UPGRADE))
SqlPerformScript(sch.Upgrade());
if(sch.ScriptChanged(SqlSchema::ATTRIBUTES))
SqlPerformScript(sch.Attributes());
sch.SaveNormal();
#endif
PersonsDlg().Run();
}
SqlCtrls.lay
LAYOUT(PersonLayout, 260, 116)
ITEM(Label, dv___0, SetLabel(t_("First Name")).LeftPosZ(8, 60).TopPosZ(8, 21))
ITEM(EditString, name, LeftPosZ(72, 180).TopPosZ(8, 19))
ITEM(Label, dv___2, SetLabel(t_("Last Name")).LeftPosZ(8, 60).TopPosZ(32, 21))
ITEM(EditString, lastname, LeftPosZ(72, 180).TopPosZ(32, 19))
ITEM(Label, dv___4, SetLabel(t_("Age")).LeftPosZ(8, 60).TopPosZ(56, 20))
ITEM(EditInt, age, LeftPosZ(72, 64).TopPosZ(56, 19))
ITEM(Button, ok, SetLabel(t_("OK")).LeftPosZ(188, 64).TopPosZ(84, 24))
ITEM(Button, cancel, SetLabel(t_("Storno")).LeftPosZ(116, 64).TopPosZ(84, 24))
END_LAYOUT
LAYOUT(PersonsLayout, 556, 324)
ITEM(SqlArray, list, LeftPosZ(4, 548).TopPosZ(4, 288))
ITEM(Button, edit, SetLabel(t_("Edit")).LeftPosZ(76, 64).TopPosZ(296, 24))
ITEM(Button, remove, SetLabel(t_("Remove")).LeftPosZ(148, 64).TopPosZ(296, 24))
ITEM(Button, create, SetLabel(t_("New")).LeftPosZ(4, 64).TopPosZ(296, 24))
END_LAYOUT
Model.sch
TABLE_(PERSON)
INT_ (ID) PRIMARY_KEY
STRING_ (NAME, 200)
STRING_ (LASTNAME, 200)
INT_ (AGE)
END_TABLE
|