class Option : public Pusher
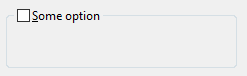
Widget providing the selection of 2 or alternatively 3 states (true, false, Null). Value of Option is either 0, 1, or Null. When setting Value to Option, string "1" is interpreted as true state, other non-Null strings as false. Depending on whether Option is in NotNull mode, Null is interpreted as false or Null state. 0 is always interpreted as false, Null number as either Null (NotNull mode) or false (NoNotNull mode), other numbers as true. The control may be also known under checbox name.
Derived from Pusher.
Below is the exemplary usage of Option control with the detailed commentary:
#include <CtrlLib/CtrlLib.h>
using namespace Upp;
struct MyAppWindow : TopWindow {
Option option;
MyAppWindow() {
SetRect(0, 0, 640, 480);
Add(option.SetLabel("Option").LeftPosZ(20).TopPosZ(20));
// By default option is disabled - to change that
// let's enable it using Set() method.
//
// Alternatives:
// - option.Set(true);
// - option.Set(static_cast<int>(true));
// - option.SetData("1");
option.Set(1);
// Let's react when option value will be change by the user.
option << [=] {
Title(Format("Option (Check-box) state changed to %d", option.Get()));
};
}
};
GUI_APP_MAIN
{
MyAppWindow app;
app.Run();
if(app.option)
PromptOK("The option was enabled!");
else
PromptOK("The option was disabled!");
// Alternatives:
// - app.option == 1 // enabled
// - app.option == 0 // disabled
// - app.option == static_cast<int>(Null) // mixed (tri-state mode)
//
// - bool enabled = static_cast<bool>(app.option);
//
// Also to obtain value Get() method could be used:
// - app.option.Get() == 1
// - ...
}
Option()
Initializes Option into NotNull, 2-state mode, false value and standard appearance.
~Option()
Default destructor.
Option& Set(int b)
Sets Option to the specified state (0, 1 or Null).
int Get() const
|
Return value |
Current state of Option. |
operator int() const
void operator=(int b)
Same as Set(b).
void EnableBox(bool b)
Enables/disables all widgets that are children of Option's parent and are intersecting Option rectangle.
void EnableBox()
Enables/disables all widgets that are children of Option's parent and are intersecting Option rectangle based on the status of the Option.
Option& BlackEdge(bool b = true)
Activates visual appearance suitable for placing Option on white background (SColorPaper), like in list, as opposed to default representation suitable for placing it on dialog (SColorFace).
|
Return value |
*this for chaining. |
bool IsBlackEdge() const
Returns true if BlackEdge is active.
Option& SwitchImage(bool b = true)
Activates visual representation that paints the Option with the Switch appearance.
bool IsSwitchImage() const
Returns true if SwitchImage is active.
Option& ThreeState(bool b = true)
Activates three-state mode. Also activates NoNotNull mode.
bool IsThreeState() const
Returns true if ThreeState is active.
Option& NotNull(bool nn = true)
Activates NotNull mode - Null Value assigned to Option (via SetData) is interpreted as false.
Option& NoNotNull()
Activates NoNotNull mode - Null Value assigned to Option is interpreted as Null.
|
Return value |
*this for chaining. |
bool IsNotNull() const
Returns true if NotNull is active.
Option& SetColor(Color c)
Sets the color of text of Option label. Setting Null restores the default color.
Option& Box(bool b = true)
Changes the visual representation to "checked box".
Option& AutoBox(bool b = true)
Calls Box(b) and activates mode where all widgets that are children of Option's parent and are intersecting Option rectangle are enabled / disabled based on the status of the Option.
Option& ShowLabel(bool b = true)
Toggles the display of the option's label.
bool IsShowLabel() const
Returns true if ShowLabel is active.
class OptionBox : public Option
Option with Box modifier active.
|